mirror of
https://github.com/arduino/arduino-ide.git
synced 2025-04-27 16:57:19 +00:00
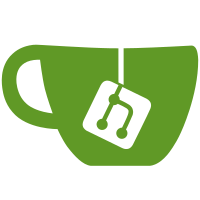
* Rebuild gRPC protocol interfaces * Implement methods to get user fields for board/port combination * Implement dialog to input board user fields * Add configure and upload step when uploading to board requiring user fields * Disable Sketch > Configure and Upload menu if board doesn't support user fields * Fix serial upload not working with all boards * Update i18n source file * fix user fields UI * regenerate cli protocol * fix localisation * check if user fields are empty Co-authored-by: Alberto Iannaccone <a.iannaccone@arduino.cc>
62 lines
1.7 KiB
TypeScript
62 lines
1.7 KiB
TypeScript
import { BoardUserField } from '.';
|
|
import { Port } from '../../common/protocol/boards-service';
|
|
import { Programmer } from './boards-service';
|
|
|
|
export const CompilerWarningLiterals = [
|
|
'None',
|
|
'Default',
|
|
'More',
|
|
'All',
|
|
] as const;
|
|
export type CompilerWarnings = typeof CompilerWarningLiterals[number];
|
|
|
|
export const CoreServicePath = '/services/core-service';
|
|
export const CoreService = Symbol('CoreService');
|
|
export interface CoreService {
|
|
compile(
|
|
options: CoreService.Compile.Options &
|
|
Readonly<{
|
|
exportBinaries?: boolean;
|
|
compilerWarnings?: CompilerWarnings;
|
|
}>
|
|
): Promise<void>;
|
|
upload(options: CoreService.Upload.Options): Promise<void>;
|
|
uploadUsingProgrammer(options: CoreService.Upload.Options): Promise<void>;
|
|
burnBootloader(options: CoreService.Bootloader.Options): Promise<void>;
|
|
isUploading(): Promise<boolean>;
|
|
}
|
|
|
|
export namespace CoreService {
|
|
export namespace Compile {
|
|
export interface Options {
|
|
/**
|
|
* `file` URI to the sketch folder.
|
|
*/
|
|
readonly sketchUri: string;
|
|
readonly fqbn?: string | undefined;
|
|
readonly optimizeForDebug: boolean;
|
|
readonly verbose: boolean;
|
|
readonly sourceOverride: Record<string, string>;
|
|
}
|
|
}
|
|
|
|
export namespace Upload {
|
|
export interface Options extends Compile.Options {
|
|
readonly port?: Port | undefined;
|
|
readonly programmer?: Programmer | undefined;
|
|
readonly verify: boolean;
|
|
readonly userFields: BoardUserField[];
|
|
}
|
|
}
|
|
|
|
export namespace Bootloader {
|
|
export interface Options {
|
|
readonly fqbn?: string | undefined;
|
|
readonly port?: Port | undefined;
|
|
readonly programmer?: Programmer | undefined;
|
|
readonly verbose: boolean;
|
|
readonly verify: boolean;
|
|
}
|
|
}
|
|
}
|