mirror of
https://github.com/arduino/arduino-ide.git
synced 2025-07-05 10:26:33 +00:00
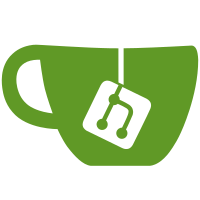
* make dialogs scroll when scaling up the UI * add unit of measure to settings step input * wrap settings dialog items when scaling up the UI * fix dialogs width when scaling up the UI * rework board config UI to make it scale up better * refactor ide updater dialog: move buttons outside the dialog content * refactor ide updater dialog: clean-up code and rename events * fix board config dialog title case and and remove double ellipsis
48 lines
2.0 KiB
TypeScript
48 lines
2.0 KiB
TypeScript
import { Emitter } from '@theia/core';
|
|
import { injectable } from '@theia/core/shared/inversify';
|
|
import { UpdateInfo, ProgressInfo } from 'electron-updater';
|
|
import { IDEUpdaterClient } from '../../common/protocol/ide-updater';
|
|
|
|
@injectable()
|
|
export class IDEUpdaterClientImpl implements IDEUpdaterClient {
|
|
protected readonly onUpdaterDidFailEmitter = new Emitter<Error>();
|
|
protected readonly onUpdaterDidCheckForUpdateEmitter = new Emitter<void>();
|
|
protected readonly onUpdaterDidFindUpdateAvailableEmitter =
|
|
new Emitter<UpdateInfo>();
|
|
protected readonly onUpdaterDidNotFindUpdateAvailableEmitter =
|
|
new Emitter<UpdateInfo>();
|
|
protected readonly onDownloadProgressDidChangeEmitter =
|
|
new Emitter<ProgressInfo>();
|
|
protected readonly onDownloadDidFinishEmitter = new Emitter<UpdateInfo>();
|
|
|
|
readonly onUpdaterDidFail = this.onUpdaterDidFailEmitter.event;
|
|
readonly onUpdaterDidCheckForUpdate =
|
|
this.onUpdaterDidCheckForUpdateEmitter.event;
|
|
readonly onUpdaterDidFindUpdateAvailable =
|
|
this.onUpdaterDidFindUpdateAvailableEmitter.event;
|
|
readonly onUpdaterDidNotFindUpdateAvailable =
|
|
this.onUpdaterDidNotFindUpdateAvailableEmitter.event;
|
|
readonly onDownloadProgressDidChange =
|
|
this.onDownloadProgressDidChangeEmitter.event;
|
|
readonly onDownloadDidFinish = this.onDownloadDidFinishEmitter.event;
|
|
|
|
notifyUpdaterFailed(message: Error): void {
|
|
this.onUpdaterDidFailEmitter.fire(message);
|
|
}
|
|
notifyCheckedForUpdate(message: void): void {
|
|
this.onUpdaterDidCheckForUpdateEmitter.fire(message);
|
|
}
|
|
notifyUpdateAvailableFound(message: UpdateInfo): void {
|
|
this.onUpdaterDidFindUpdateAvailableEmitter.fire(message);
|
|
}
|
|
notifyUpdateAvailableNotFound(message: UpdateInfo): void {
|
|
this.onUpdaterDidNotFindUpdateAvailableEmitter.fire(message);
|
|
}
|
|
notifyDownloadProgressChanged(message: ProgressInfo): void {
|
|
this.onDownloadProgressDidChangeEmitter.fire(message);
|
|
}
|
|
notifyDownloadFinished(message: UpdateInfo): void {
|
|
this.onDownloadDidFinishEmitter.fire(message);
|
|
}
|
|
}
|