mirror of
https://github.com/arduino/arduino-ide.git
synced 2025-07-11 21:36:33 +00:00
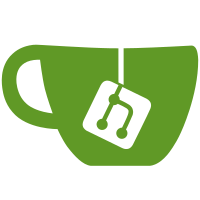
Co-authored-by: Mark Sujew <mark.sujew@typefox.io> Co-authored-by: Akos Kitta <a.kitta@arduino.cc> Signed-off-by: Akos Kitta <a.kitta@arduino.cc>
38 lines
1.1 KiB
TypeScript
38 lines
1.1 KiB
TypeScript
import { injectable, inject } from '@theia/core/shared/inversify';
|
|
import { StorageService } from '@theia/core/lib/browser/storage-service';
|
|
import {
|
|
Command,
|
|
CommandContribution,
|
|
CommandRegistry,
|
|
} from '@theia/core/lib/common/command';
|
|
|
|
/**
|
|
* This is a workaround to break cycles in the dependency injection. Provides commands for `setData` and `getData`.
|
|
*/
|
|
@injectable()
|
|
export class StorageWrapper implements CommandContribution {
|
|
@inject(StorageService)
|
|
protected storageService: StorageService;
|
|
|
|
registerCommands(commands: CommandRegistry): void {
|
|
commands.registerCommand(StorageWrapper.Commands.GET_DATA, {
|
|
execute: (key: string, defaultValue?: any) =>
|
|
this.storageService.getData(key, defaultValue),
|
|
});
|
|
commands.registerCommand(StorageWrapper.Commands.SET_DATA, {
|
|
execute: (key: string, value: any) =>
|
|
this.storageService.setData(key, value),
|
|
});
|
|
}
|
|
}
|
|
export namespace StorageWrapper {
|
|
export namespace Commands {
|
|
export const SET_DATA: Command = {
|
|
id: 'arduino-store-wrapper-set',
|
|
};
|
|
export const GET_DATA: Command = {
|
|
id: 'arduino-store-wrapper-get',
|
|
};
|
|
}
|
|
}
|