mirror of
https://github.com/home-assistant/core.git
synced 2025-04-25 01:38:02 +00:00
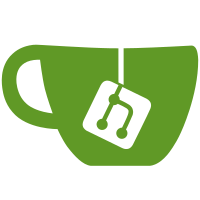
* Add Lektrico Integration * Make the changes proposed by Lash-L: new coordinator.py, new entity.py; use: translation_key, last_update_sucess, PlatformNotReady; remove: global variables * Replace FlowResult with ConfigFlowResult and add tests. * Remove unused lines. * Remove Options from condif_flow * Fix ruff and mypy. * Fix CODEOWNERS. * Run python3 -m script.hassfest. * Correct rebase mistake. * Make modifications suggested by emontnemery. * Add pytest fixtures. * Remove meaningless patches. * Update .coveragerc * Replace CONF_FRIENDLY_NAME with CONF_NAME. * Remove underscores. * Update tests. * Update test file with is and no config_entries. . * Set serial_number in DeviceInfo and add return type of the async_update_data to DataUpdateCoordinator. * Use suggested_unit_of_measurement for KILO_WATT and replace Any in value_fn (sensor file). * Add device class duration to charging_time sensor. * Change raising PlatformNotReady to raising IntegrationError. * Test the unique id of the entry. * Rename PF Lx with Power factor Lx and remove PF from strings.json. * Remove comment. * Make state and limit reason sensors to be enum sensors. * Use result variable to check unique_id in test. * Remove CONF_NAME from entry and __init__ from LektricoFlowHandler. * Remove session parameter from LektricoDeviceDataUpdateCoordinator. * Use config_entry: ConfigEntry in coordinator. * Replace Connected,NeedAuth with Waiting for Authentication. * Use lektricowifi 0.0.29. * Use lektricowifi 0.0.39 * Use lektricowifi 0.0.40 * Use lektricowifi 0.0.41 * Replace hass.data with entry.runtime_data * Delete .coveragerc * Restructure the user step * Fix tests * Add returned value of _async_update_data to class DataUpdateCoordinator * Use hw_version at DeviceInfo * Remove a variable * Use StateType * Replace friendly_name with device_name * Use sentence case in translation strings * Uncomment and fix test_discovered_zeroconf * Add type LektricoConfigEntry * Remove commented code * Remove the type of coordinator in sensor async_setup_entry * Make zeroconf test end in ABORT, not FORM * Remove all async_block_till_done from tests * End test_user_setup_device_offline with CREATE_ENTRY * Patch the full Device * Add snapshot tests * Overwrite the type LektricoSensorEntityDescription outside of the constructor * Test separate already_configured for zeroconf --------- Co-authored-by: mihaela.tarjoianu <mihaela.tarjoianu@scada.ro> Co-authored-by: Erik Montnemery <erik@montnemery.com>
93 lines
2.5 KiB
Python
93 lines
2.5 KiB
Python
"""Fixtures for Lektrico Charging Station integration tests."""
|
|
|
|
from collections.abc import Generator
|
|
from ipaddress import ip_address
|
|
import json
|
|
from unittest.mock import AsyncMock, patch
|
|
|
|
import pytest
|
|
|
|
from homeassistant.components.lektrico.const import DOMAIN
|
|
from homeassistant.components.zeroconf import ZeroconfServiceInfo
|
|
from homeassistant.const import (
|
|
ATTR_HW_VERSION,
|
|
ATTR_SERIAL_NUMBER,
|
|
CONF_HOST,
|
|
CONF_TYPE,
|
|
)
|
|
|
|
from tests.common import MockConfigEntry, load_fixture
|
|
|
|
MOCKED_DEVICE_IP_ADDRESS = "192.168.100.10"
|
|
MOCKED_DEVICE_SERIAL_NUMBER = "500006"
|
|
MOCKED_DEVICE_TYPE = "1p7k"
|
|
MOCKED_DEVICE_BOARD_REV = "B"
|
|
|
|
MOCKED_DEVICE_ZC_NAME = "Lektrico-1p7k-500006._http._tcp"
|
|
MOCKED_DEVICE_ZC_TYPE = "_http._tcp.local."
|
|
MOCKED_DEVICE_ZEROCONF_DATA = ZeroconfServiceInfo(
|
|
ip_address=ip_address(MOCKED_DEVICE_IP_ADDRESS),
|
|
ip_addresses=[ip_address(MOCKED_DEVICE_IP_ADDRESS)],
|
|
hostname=f"{MOCKED_DEVICE_ZC_NAME.lower()}.local.",
|
|
port=80,
|
|
type=MOCKED_DEVICE_ZC_TYPE,
|
|
name=MOCKED_DEVICE_ZC_NAME,
|
|
properties={
|
|
"id": "1p7k_500006",
|
|
"fw_id": "20230109-124642/v1.22-36-g56a3edd-develop-dirty",
|
|
},
|
|
)
|
|
|
|
|
|
@pytest.fixture
|
|
def mock_device() -> Generator[AsyncMock]:
|
|
"""Mock a Lektrico device."""
|
|
with (
|
|
patch(
|
|
"homeassistant.components.lektrico.Device",
|
|
autospec=True,
|
|
) as mock_device,
|
|
patch(
|
|
"homeassistant.components.lektrico.config_flow.Device",
|
|
new=mock_device,
|
|
),
|
|
patch(
|
|
"homeassistant.components.lektrico.coordinator.Device",
|
|
new=mock_device,
|
|
),
|
|
):
|
|
device = mock_device.return_value
|
|
|
|
device.device_config.return_value = json.loads(
|
|
load_fixture("get_config.json", DOMAIN)
|
|
)
|
|
device.device_info.return_value = json.loads(
|
|
load_fixture("get_info.json", DOMAIN)
|
|
)
|
|
|
|
yield device
|
|
|
|
|
|
@pytest.fixture
|
|
def mock_setup_entry() -> Generator[AsyncMock]:
|
|
"""Mock setup entry."""
|
|
with patch(
|
|
"homeassistant.components.lektrico.async_setup_entry", return_value=True
|
|
) as mock_setup_entry:
|
|
yield mock_setup_entry
|
|
|
|
|
|
@pytest.fixture
|
|
def mock_config_entry() -> MockConfigEntry:
|
|
"""Mock a config entry."""
|
|
return MockConfigEntry(
|
|
domain=DOMAIN,
|
|
data={
|
|
CONF_HOST: MOCKED_DEVICE_IP_ADDRESS,
|
|
CONF_TYPE: MOCKED_DEVICE_TYPE,
|
|
ATTR_SERIAL_NUMBER: MOCKED_DEVICE_SERIAL_NUMBER,
|
|
ATTR_HW_VERSION: "B",
|
|
},
|
|
unique_id=MOCKED_DEVICE_SERIAL_NUMBER,
|
|
)
|