mirror of
https://github.com/home-assistant/core.git
synced 2025-05-13 10:29:14 +00:00
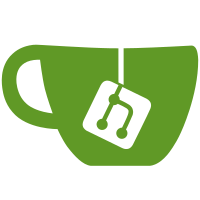
* First draft * Generate device id * No obscure registry * Dont store config_entry_id in device * Storage * Small mistake on rebase * Do storage more like entity registry * Improve device identification * Add tests * Remove deconz device support from PR * Fix hound comments, voff! * Fix comments and clean up * Fix proper indentation * Fix pydoc issues * Fix mochad component to not use self.device * Fix mochad light platform to not use self.device * Fix TankUtilitySensor to not use self.device * Fix Soundtouch to not use self.device * Fix Plex to not use self.device * Fix Emby to not use self.device * Fix Heatmiser to not use self.device * Fix Wemo lights to not use self.device * Fix Lifx to not use self.device * Fix Radiotherm to not use self.device * Fix Juicenet to not use self.device * Fix Qwikswitch to not use self.device * Fix Xiaomi miio to not use self.device * Fix Nest to not use self.device * Fix Tellduslive to not use self.device * Fix Knx to not use self.device * Clean up a small mistake in soundtouch * Fix comment from Ballob * Fix bad indentation * Fix indentatin * Lint * Remove unused variable * Lint
75 lines
1.8 KiB
Python
75 lines
1.8 KiB
Python
"""
|
|
Support for Juicenet cloud.
|
|
|
|
For more details about this component, please refer to the documentation at
|
|
https://home-assistant.io/components/juicenet
|
|
"""
|
|
|
|
import logging
|
|
|
|
import voluptuous as vol
|
|
|
|
from homeassistant.helpers import discovery
|
|
from homeassistant.const import CONF_ACCESS_TOKEN
|
|
from homeassistant.helpers.entity import Entity
|
|
import homeassistant.helpers.config_validation as cv
|
|
|
|
REQUIREMENTS = ['python-juicenet==0.0.5']
|
|
|
|
_LOGGER = logging.getLogger(__name__)
|
|
|
|
DOMAIN = 'juicenet'
|
|
|
|
CONFIG_SCHEMA = vol.Schema({
|
|
DOMAIN: vol.Schema({
|
|
vol.Required(CONF_ACCESS_TOKEN): cv.string
|
|
})
|
|
}, extra=vol.ALLOW_EXTRA)
|
|
|
|
|
|
def setup(hass, config):
|
|
"""Set up the Juicenet component."""
|
|
import pyjuicenet
|
|
|
|
hass.data[DOMAIN] = {}
|
|
|
|
access_token = config[DOMAIN].get(CONF_ACCESS_TOKEN)
|
|
hass.data[DOMAIN]['api'] = pyjuicenet.Api(access_token)
|
|
|
|
discovery.load_platform(hass, 'sensor', DOMAIN, {}, config)
|
|
return True
|
|
|
|
|
|
class JuicenetDevice(Entity):
|
|
"""Represent a base Juicenet device."""
|
|
|
|
def __init__(self, device, sensor_type, hass):
|
|
"""Initialise the sensor."""
|
|
self.hass = hass
|
|
self._device = device
|
|
self.type = sensor_type
|
|
|
|
@property
|
|
def name(self):
|
|
"""Return the name of the device."""
|
|
return self._device.name()
|
|
|
|
def update(self):
|
|
"""Update state of the device."""
|
|
self._device.update_state()
|
|
|
|
@property
|
|
def _manufacturer_device_id(self):
|
|
"""Return the manufacturer device id."""
|
|
return self._device.id()
|
|
|
|
@property
|
|
def _token(self):
|
|
"""Return the device API token."""
|
|
return self._device.token()
|
|
|
|
@property
|
|
def unique_id(self):
|
|
"""Return a unique ID."""
|
|
return "{}-{}".format(self._device.id(), self.type)
|