mirror of
https://github.com/home-assistant/core.git
synced 2025-07-13 08:17:08 +00:00
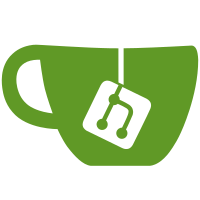
* Implemented coordinator (for Cloud integration) * Optimized coordinator updates * Finalizing * Running ruff and ruff format * Raise error if trying to instantiate coordinator for a AdaxLocal config * Re-added data-handler for AdaxLocal integrations * Added a coordinator for Local integrations * mypy warnings * Update homeassistant/components/adax/manifest.json Co-authored-by: Daniel Hjelseth Høyer <mail@dahoiv.net> * Resolve mypy issues * PR comments - Explicit passing of config_entry to Coordinator base type - Avoid duplicate storing of Coordinator data. Instead use self.data - Remove try-catch and wrapping to UpdateFailed in _async_update_data - Custom ConfigEntry type for passing coordinator via entry.runtime_data * When changing HVAC_MODE update data via Coordinator to optimize * Apply already loaded data for Climate entity directly in __init__ * Moved SCAN_INTERVAL into const.py * Removed logging statements * Remove unnecessary get_rooms() / get_status() functions * Resolvning mypy issues * Adding tests for coordinators * Resolving review comments by joostlek * Setup of Cloud devices with device_id * Implement Climate tests for Adax * Implementing assertions of UNAVAILABLE state * Removed no longer needed method * Apply suggestions from code review Co-authored-by: Joost Lekkerkerker <joostlek@outlook.com> * Mock Adax class instead of individual methods * Mock config entry via fixture * Load config entry data from .json fixture * Hard code config_entry_data instead of .json file * Removed obsolete .json-files * Fix * Fix --------- Co-authored-by: Daniel Hjelseth Høyer <mail@dahoiv.net> Co-authored-by: Joost Lekkerkerker <joostlek@outlook.com>
86 lines
2.7 KiB
Python
86 lines
2.7 KiB
Python
"""Test Adax climate entity."""
|
|
|
|
from homeassistant.components.adax.const import SCAN_INTERVAL
|
|
from homeassistant.components.climate import ATTR_CURRENT_TEMPERATURE, HVACMode
|
|
from homeassistant.const import ATTR_TEMPERATURE, STATE_UNAVAILABLE, Platform
|
|
from homeassistant.core import HomeAssistant
|
|
|
|
from . import setup_integration
|
|
from .conftest import CLOUD_DEVICE_DATA, LOCAL_DEVICE_DATA
|
|
|
|
from tests.common import AsyncMock, MockConfigEntry, async_fire_time_changed
|
|
from tests.test_setup import FrozenDateTimeFactory
|
|
|
|
|
|
async def test_climate_cloud(
|
|
hass: HomeAssistant,
|
|
freezer: FrozenDateTimeFactory,
|
|
mock_cloud_config_entry: MockConfigEntry,
|
|
mock_adax_cloud: AsyncMock,
|
|
) -> None:
|
|
"""Test states of the (cloud) Climate entity."""
|
|
await setup_integration(hass, mock_cloud_config_entry)
|
|
mock_adax_cloud.get_rooms.assert_called_once()
|
|
|
|
assert len(hass.states.async_entity_ids(Platform.CLIMATE)) == 1
|
|
entity_id = hass.states.async_entity_ids(Platform.CLIMATE)[0]
|
|
|
|
state = hass.states.get(entity_id)
|
|
|
|
assert state
|
|
assert state.state == HVACMode.HEAT
|
|
assert (
|
|
state.attributes[ATTR_TEMPERATURE] == CLOUD_DEVICE_DATA[0]["targetTemperature"]
|
|
)
|
|
assert (
|
|
state.attributes[ATTR_CURRENT_TEMPERATURE]
|
|
== CLOUD_DEVICE_DATA[0]["temperature"]
|
|
)
|
|
|
|
mock_adax_cloud.get_rooms.side_effect = Exception()
|
|
freezer.tick(SCAN_INTERVAL)
|
|
async_fire_time_changed(hass)
|
|
await hass.async_block_till_done()
|
|
|
|
state = hass.states.get(entity_id)
|
|
assert state
|
|
assert state.state == STATE_UNAVAILABLE
|
|
|
|
|
|
async def test_climate_local(
|
|
hass: HomeAssistant,
|
|
freezer: FrozenDateTimeFactory,
|
|
mock_local_config_entry: MockConfigEntry,
|
|
mock_adax_local: AsyncMock,
|
|
) -> None:
|
|
"""Test states of the (local) Climate entity."""
|
|
await setup_integration(hass, mock_local_config_entry)
|
|
mock_adax_local.get_status.assert_called_once()
|
|
|
|
assert len(hass.states.async_entity_ids(Platform.CLIMATE)) == 1
|
|
entity_id = hass.states.async_entity_ids(Platform.CLIMATE)[0]
|
|
|
|
freezer.tick(SCAN_INTERVAL)
|
|
async_fire_time_changed(hass)
|
|
await hass.async_block_till_done()
|
|
|
|
state = hass.states.get(entity_id)
|
|
assert state
|
|
assert state.state == HVACMode.HEAT
|
|
assert (
|
|
state.attributes[ATTR_TEMPERATURE] == (LOCAL_DEVICE_DATA["target_temperature"])
|
|
)
|
|
assert (
|
|
state.attributes[ATTR_CURRENT_TEMPERATURE]
|
|
== (LOCAL_DEVICE_DATA["current_temperature"])
|
|
)
|
|
|
|
mock_adax_local.get_status.side_effect = Exception()
|
|
freezer.tick(SCAN_INTERVAL)
|
|
async_fire_time_changed(hass)
|
|
await hass.async_block_till_done()
|
|
|
|
state = hass.states.get(entity_id)
|
|
assert state
|
|
assert state.state == STATE_UNAVAILABLE
|