mirror of
https://github.com/home-assistant/core.git
synced 2025-05-13 18:39:14 +00:00
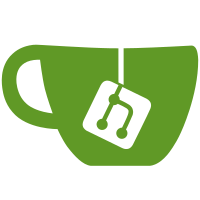
* Add entity tests * Add __init__ tests * Cleanup * Rename mock_motionmount_config_flow to mock_motionmount * Remove unneeded PropertyMock * Set defaults on mock_motionmount * Test proper device is created * Check whether proper device is created from test_init.py, also without mac * Find callback and use that to update name
130 lines
4.0 KiB
Python
130 lines
4.0 KiB
Python
"""Tests for the MotionMount init."""
|
|
|
|
from unittest.mock import MagicMock
|
|
|
|
from homeassistant.components.motionmount import DOMAIN
|
|
from homeassistant.config_entries import SOURCE_REAUTH, ConfigEntryState
|
|
from homeassistant.core import HomeAssistant
|
|
from homeassistant.helpers import device_registry as dr
|
|
from homeassistant.helpers.device_registry import format_mac
|
|
|
|
from tests.common import MockConfigEntry
|
|
|
|
|
|
async def test_setup_entry_with_mac(
|
|
hass: HomeAssistant,
|
|
device_registry: dr.DeviceRegistry,
|
|
mock_config_entry: MockConfigEntry,
|
|
mock_motionmount: MagicMock,
|
|
) -> None:
|
|
"""Tests the state attributes."""
|
|
mock_config_entry.add_to_hass(hass)
|
|
|
|
assert await hass.config_entries.async_setup(mock_config_entry.entry_id)
|
|
|
|
assert mock_config_entry.state is ConfigEntryState.LOADED
|
|
|
|
mac = format_mac(mock_motionmount.mac.hex())
|
|
device = device_registry.async_get_device(
|
|
connections={(dr.CONNECTION_NETWORK_MAC, mac)}
|
|
)
|
|
assert device
|
|
assert device.name == mock_config_entry.title
|
|
|
|
|
|
async def test_setup_entry_without_mac(
|
|
hass: HomeAssistant,
|
|
device_registry: dr.DeviceRegistry,
|
|
mock_config_entry: MockConfigEntry,
|
|
mock_motionmount: MagicMock,
|
|
) -> None:
|
|
"""Tests the state attributes."""
|
|
mock_config_entry.add_to_hass(hass)
|
|
|
|
mock_motionmount.mac = b"\x00\x00\x00\x00\x00\x00"
|
|
|
|
assert await hass.config_entries.async_setup(mock_config_entry.entry_id)
|
|
|
|
assert mock_config_entry.state is ConfigEntryState.LOADED
|
|
|
|
device = device_registry.async_get_device(
|
|
identifiers={(DOMAIN, mock_config_entry.entry_id)}
|
|
)
|
|
assert device
|
|
assert device.name == mock_config_entry.title
|
|
|
|
|
|
async def test_setup_entry_failed_connect(
|
|
hass: HomeAssistant,
|
|
mock_config_entry: MockConfigEntry,
|
|
mock_motionmount: MagicMock,
|
|
) -> None:
|
|
"""Tests the state attributes."""
|
|
mock_config_entry.add_to_hass(hass)
|
|
|
|
mock_motionmount.connect.side_effect = TimeoutError()
|
|
assert not await hass.config_entries.async_setup(mock_config_entry.entry_id)
|
|
|
|
assert mock_config_entry.state is ConfigEntryState.SETUP_RETRY
|
|
|
|
|
|
async def test_setup_entry_wrong_device(
|
|
hass: HomeAssistant,
|
|
mock_config_entry: MockConfigEntry,
|
|
mock_motionmount: MagicMock,
|
|
) -> None:
|
|
"""Tests the state attributes."""
|
|
mock_config_entry.add_to_hass(hass)
|
|
|
|
mock_motionmount.mac = b"\x00\x00\x00\x00\x00\x01"
|
|
assert not await hass.config_entries.async_setup(mock_config_entry.entry_id)
|
|
|
|
assert mock_config_entry.state is ConfigEntryState.SETUP_RETRY
|
|
|
|
|
|
async def test_setup_entry_no_pin(
|
|
hass: HomeAssistant,
|
|
mock_config_entry: MockConfigEntry,
|
|
mock_motionmount: MagicMock,
|
|
) -> None:
|
|
"""Tests the state attributes."""
|
|
mock_config_entry.add_to_hass(hass)
|
|
|
|
mock_motionmount.is_authenticated = False
|
|
assert not await hass.config_entries.async_setup(mock_config_entry.entry_id)
|
|
|
|
assert mock_config_entry.state is ConfigEntryState.SETUP_ERROR
|
|
assert any(mock_config_entry.async_get_active_flows(hass, sources={SOURCE_REAUTH}))
|
|
|
|
|
|
async def test_setup_entry_wrong_pin(
|
|
hass: HomeAssistant,
|
|
mock_config_entry_with_pin: MockConfigEntry,
|
|
mock_motionmount: MagicMock,
|
|
) -> None:
|
|
"""Tests the state attributes."""
|
|
mock_config_entry_with_pin.add_to_hass(hass)
|
|
|
|
mock_motionmount.is_authenticated = False
|
|
assert not await hass.config_entries.async_setup(
|
|
mock_config_entry_with_pin.entry_id
|
|
)
|
|
|
|
assert mock_config_entry_with_pin.state is ConfigEntryState.SETUP_ERROR
|
|
assert any(
|
|
mock_config_entry_with_pin.async_get_active_flows(hass, sources={SOURCE_REAUTH})
|
|
)
|
|
|
|
|
|
async def test_unload_entry(
|
|
hass: HomeAssistant,
|
|
mock_config_entry: MockConfigEntry,
|
|
mock_motionmount: MagicMock,
|
|
) -> None:
|
|
"""Test entries are unloaded correctly."""
|
|
mock_config_entry.add_to_hass(hass)
|
|
|
|
assert await hass.config_entries.async_setup(mock_config_entry.entry_id)
|
|
assert await hass.config_entries.async_unload(mock_config_entry.entry_id)
|
|
assert mock_motionmount.disconnect.call_count == 1
|