mirror of
https://github.com/home-assistant/core.git
synced 2025-04-25 17:57:55 +00:00
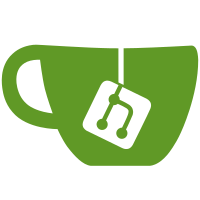
* Add remote_calendar with storage * Use coordinator and remove storage * cleanup * cleanup * remove init from config_flow * add some tests * some fixes * test-before-setup * fix error handling * remove unneeded code * fix updates * load calendar in the event loop * allow redirects * test_update_failed * tests * address review * use error from local_calendar * adress more comments * remove unique_id * add unique entity_id * add excemption * abort_entries_match * unique_id * add , * cleanup * deduplicate call * don't raise for status end de-nest * multiline * test * tests * use raise_for_status again * use respx * just use config_entry argument that already is defined * Also assert on the config entry result title and data * improve config_flow * update quality scale * address review --------- Co-authored-by: Allen Porter <allen@thebends.org>
71 lines
2.3 KiB
Python
71 lines
2.3 KiB
Python
"""Config flow for Remote Calendar integration."""
|
|
|
|
import logging
|
|
from typing import Any
|
|
|
|
from httpx import HTTPError, InvalidURL
|
|
from ical.calendar_stream import IcsCalendarStream
|
|
from ical.exceptions import CalendarParseError
|
|
import voluptuous as vol
|
|
|
|
from homeassistant.config_entries import ConfigFlow, ConfigFlowResult
|
|
from homeassistant.const import CONF_URL
|
|
from homeassistant.helpers.httpx_client import get_async_client
|
|
|
|
from .const import CONF_CALENDAR_NAME, DOMAIN
|
|
|
|
_LOGGER = logging.getLogger(__name__)
|
|
|
|
STEP_USER_DATA_SCHEMA = vol.Schema(
|
|
{
|
|
vol.Required(CONF_CALENDAR_NAME): str,
|
|
vol.Required(CONF_URL): str,
|
|
}
|
|
)
|
|
|
|
|
|
class RemoteCalendarConfigFlow(ConfigFlow, domain=DOMAIN):
|
|
"""Handle a config flow for Remote Calendar."""
|
|
|
|
VERSION = 1
|
|
|
|
async def async_step_user(
|
|
self, user_input: dict[str, Any] | None = None
|
|
) -> ConfigFlowResult:
|
|
"""Handle the initial step."""
|
|
if user_input is None:
|
|
return self.async_show_form(
|
|
step_id="user", data_schema=STEP_USER_DATA_SCHEMA
|
|
)
|
|
errors: dict = {}
|
|
_LOGGER.debug("User input: %s", user_input)
|
|
self._async_abort_entries_match(
|
|
{CONF_CALENDAR_NAME: user_input[CONF_CALENDAR_NAME]}
|
|
)
|
|
self._async_abort_entries_match({CONF_URL: user_input[CONF_URL]})
|
|
client = get_async_client(self.hass)
|
|
try:
|
|
res = await client.get(user_input[CONF_URL], follow_redirects=True)
|
|
res.raise_for_status()
|
|
except (HTTPError, InvalidURL) as err:
|
|
errors["base"] = "cannot_connect"
|
|
_LOGGER.debug("An error occurred: %s", err)
|
|
else:
|
|
try:
|
|
await self.hass.async_add_executor_job(
|
|
IcsCalendarStream.calendar_from_ics, res.text
|
|
)
|
|
except CalendarParseError as err:
|
|
errors["base"] = "invalid_ics_file"
|
|
_LOGGER.debug("Invalid .ics file: %s", err)
|
|
else:
|
|
return self.async_create_entry(
|
|
title=user_input[CONF_CALENDAR_NAME], data=user_input
|
|
)
|
|
|
|
return self.async_show_form(
|
|
step_id="user",
|
|
data_schema=STEP_USER_DATA_SCHEMA,
|
|
errors=errors,
|
|
)
|