mirror of
https://github.com/home-assistant/core.git
synced 2025-07-03 03:17:05 +00:00
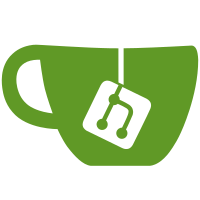
* Add remote_calendar with storage * Use coordinator and remove storage * cleanup * cleanup * remove init from config_flow * add some tests * some fixes * test-before-setup * fix error handling * remove unneeded code * fix updates * load calendar in the event loop * allow redirects * test_update_failed * tests * address review * use error from local_calendar * adress more comments * remove unique_id * add unique entity_id * add excemption * abort_entries_match * unique_id * add , * cleanup * deduplicate call * don't raise for status end de-nest * multiline * test * tests * use raise_for_status again * use respx * just use config_entry argument that already is defined * Also assert on the config entry result title and data * improve config_flow * update quality scale * address review --------- Co-authored-by: Allen Porter <allen@thebends.org>
74 lines
2.0 KiB
Python
74 lines
2.0 KiB
Python
"""Tests for init platform of Remote Calendar."""
|
|
|
|
from httpx import ConnectError, Response, UnsupportedProtocol
|
|
import pytest
|
|
import respx
|
|
|
|
from homeassistant.config_entries import ConfigEntryState
|
|
from homeassistant.const import STATE_OFF
|
|
from homeassistant.core import HomeAssistant
|
|
|
|
from . import setup_integration
|
|
from .conftest import CALENDER_URL, TEST_ENTITY
|
|
|
|
from tests.common import MockConfigEntry
|
|
|
|
|
|
@respx.mock
|
|
async def test_load_unload(
|
|
hass: HomeAssistant, config_entry: MockConfigEntry, ics_content: str
|
|
) -> None:
|
|
"""Test loading and unloading a config entry."""
|
|
respx.get(CALENDER_URL).mock(
|
|
return_value=Response(
|
|
status_code=200,
|
|
text=ics_content,
|
|
)
|
|
)
|
|
await setup_integration(hass, config_entry)
|
|
assert config_entry.state is ConfigEntryState.LOADED
|
|
|
|
state = hass.states.get(TEST_ENTITY)
|
|
assert state
|
|
assert state.state == STATE_OFF
|
|
|
|
await hass.config_entries.async_unload(config_entry.entry_id)
|
|
await hass.async_block_till_done()
|
|
|
|
assert config_entry.state is ConfigEntryState.NOT_LOADED
|
|
|
|
|
|
@respx.mock
|
|
async def test_raise_for_status(
|
|
hass: HomeAssistant,
|
|
config_entry: MockConfigEntry,
|
|
) -> None:
|
|
"""Test update failed using respx to simulate HTTP exceptions."""
|
|
respx.get(CALENDER_URL).mock(
|
|
return_value=Response(
|
|
status_code=403,
|
|
)
|
|
)
|
|
await setup_integration(hass, config_entry)
|
|
assert config_entry.state is ConfigEntryState.SETUP_RETRY
|
|
|
|
|
|
@pytest.mark.parametrize(
|
|
"side_effect",
|
|
[
|
|
ConnectError("Connection failed"),
|
|
UnsupportedProtocol("Unsupported protocol"),
|
|
ValueError("Invalid response"),
|
|
],
|
|
)
|
|
@respx.mock
|
|
async def test_update_failed(
|
|
hass: HomeAssistant,
|
|
config_entry: MockConfigEntry,
|
|
side_effect: Exception,
|
|
) -> None:
|
|
"""Test update failed using respx to simulate different exceptions."""
|
|
respx.get(CALENDER_URL).mock(side_effect=side_effect)
|
|
await setup_integration(hass, config_entry)
|
|
assert config_entry.state is ConfigEntryState.SETUP_RETRY
|