mirror of
https://github.com/home-assistant/core.git
synced 2025-04-25 17:57:55 +00:00
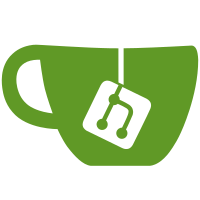
* chore: Refactor BSBLanUpdateCoordinator to improve code readability and maintainability * feat: Add BSBLan integration models This commit adds the models for the BSB-Lan integration. It includes a dataclass for the BSBLanCoordinatorData, which stores the state and sensor information. * refactor: Update BSBLANClimate class to use DataUpdateCoordinator without specifying the State type * chore: Remove unused Sensor import in BSBLan models * feat: Refactor BSBLanEntity to use CoordinatorEntity The BSBLanEntity class has been refactored to inherit from the CoordinatorEntity class, which provides better integration with the update coordinator. This change improves code readability and maintainability. * refactor: Remove unused config_entry variable in BSBLanUpdateCoordinator * refactor: Update BSBLANClimate class to use DataUpdateCoordinator Refactor the BSBLANClimate class to use the Coordinator of the entity * refactor: Update tests to use the new structure * fix coverage it should be the same as before * refactor: moved dataclass BSBLanCoordinatorData * use the data class inside init * refactor: Remove unused config_entry variable in BSBLanUpdateCoordinator * refactor: use BSBLanData from init * remove entry data from diagnostics * fix: add random interval back * refactor: Simplify coordinator_data assignment in async_get_config_entry_diagnostics * revert back to original except dataclass import * revert: Add MAC address back to device info in BSBLanEntity
65 lines
2.1 KiB
Python
65 lines
2.1 KiB
Python
"""DataUpdateCoordinator for the BSB-Lan integration."""
|
|
|
|
from dataclasses import dataclass
|
|
from datetime import timedelta
|
|
from random import randint
|
|
|
|
from bsblan import BSBLAN, BSBLANConnectionError, State
|
|
|
|
from homeassistant.config_entries import ConfigEntry
|
|
from homeassistant.const import CONF_HOST
|
|
from homeassistant.core import HomeAssistant
|
|
from homeassistant.helpers.update_coordinator import DataUpdateCoordinator, UpdateFailed
|
|
|
|
from .const import DOMAIN, LOGGER, SCAN_INTERVAL
|
|
|
|
|
|
@dataclass
|
|
class BSBLanCoordinatorData:
|
|
"""BSBLan data stored in the Home Assistant data object."""
|
|
|
|
state: State
|
|
|
|
|
|
class BSBLanUpdateCoordinator(DataUpdateCoordinator[BSBLanCoordinatorData]):
|
|
"""The BSB-Lan update coordinator."""
|
|
|
|
config_entry: ConfigEntry
|
|
|
|
def __init__(
|
|
self,
|
|
hass: HomeAssistant,
|
|
config_entry: ConfigEntry,
|
|
client: BSBLAN,
|
|
) -> None:
|
|
"""Initialize the BSB-Lan coordinator."""
|
|
super().__init__(
|
|
hass,
|
|
logger=LOGGER,
|
|
name=f"{DOMAIN}_{config_entry.data[CONF_HOST]}",
|
|
update_interval=self._get_update_interval(),
|
|
)
|
|
self.client = client
|
|
|
|
def _get_update_interval(self) -> timedelta:
|
|
"""Get the update interval with a random offset.
|
|
|
|
Use the default scan interval and add a random number of seconds to avoid timeouts when
|
|
the BSB-Lan device is already/still busy retrieving data,
|
|
e.g. for MQTT or internal logging.
|
|
"""
|
|
return SCAN_INTERVAL + timedelta(seconds=randint(1, 8))
|
|
|
|
async def _async_update_data(self) -> BSBLanCoordinatorData:
|
|
"""Get state and sensor data from BSB-Lan device."""
|
|
try:
|
|
state = await self.client.state()
|
|
except BSBLANConnectionError as err:
|
|
host = self.config_entry.data[CONF_HOST] if self.config_entry else "unknown"
|
|
raise UpdateFailed(
|
|
f"Error while establishing connection with BSB-Lan device at {host}"
|
|
) from err
|
|
|
|
self.update_interval = self._get_update_interval()
|
|
return BSBLanCoordinatorData(state=state)
|