mirror of
https://github.com/home-assistant/core.git
synced 2025-07-09 22:37:11 +00:00
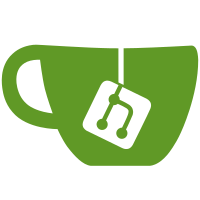
- These devices sometimes do not respond on the first try or may be subject to transient broadcast failures, or overloads. We now try discovery periodically once the integration has been loaded. - We used to try this 4x at startup, but that solution seemed to aggressive as we want to be sure we pickup the devices after startup as well since the network will likely be more calm after startup.
66 lines
2.8 KiB
Python
66 lines
2.8 KiB
Python
"""Tests for the TP-Link component."""
|
|
from __future__ import annotations
|
|
|
|
from datetime import timedelta
|
|
from unittest.mock import MagicMock, patch
|
|
|
|
from homeassistant.components import tplink
|
|
from homeassistant.components.tplink.const import DOMAIN
|
|
from homeassistant.config_entries import ConfigEntryState
|
|
from homeassistant.const import CONF_HOST, EVENT_HOMEASSISTANT_STARTED
|
|
from homeassistant.setup import async_setup_component
|
|
from homeassistant.util import dt as dt_util
|
|
|
|
from . import IP_ADDRESS, MAC_ADDRESS, _patch_discovery, _patch_single_discovery
|
|
|
|
from tests.common import MockConfigEntry, async_fire_time_changed
|
|
|
|
|
|
async def test_configuring_tplink_causes_discovery(hass):
|
|
"""Test that specifying empty config does discovery."""
|
|
with patch("homeassistant.components.tplink.Discover.discover") as discover:
|
|
discover.return_value = {MagicMock(): MagicMock()}
|
|
await async_setup_component(hass, tplink.DOMAIN, {tplink.DOMAIN: {}})
|
|
await hass.async_block_till_done()
|
|
call_count = len(discover.mock_calls)
|
|
assert discover.mock_calls
|
|
|
|
hass.bus.async_fire(EVENT_HOMEASSISTANT_STARTED)
|
|
await hass.async_block_till_done()
|
|
assert len(discover.mock_calls) == call_count * 2
|
|
|
|
async_fire_time_changed(hass, dt_util.utcnow() + timedelta(minutes=15))
|
|
await hass.async_block_till_done()
|
|
assert len(discover.mock_calls) == call_count * 3
|
|
|
|
async_fire_time_changed(hass, dt_util.utcnow() + timedelta(minutes=30))
|
|
await hass.async_block_till_done()
|
|
assert len(discover.mock_calls) == call_count * 4
|
|
|
|
|
|
async def test_config_entry_reload(hass):
|
|
"""Test that a config entry can be reloaded."""
|
|
already_migrated_config_entry = MockConfigEntry(
|
|
domain=DOMAIN, data={}, unique_id=MAC_ADDRESS
|
|
)
|
|
already_migrated_config_entry.add_to_hass(hass)
|
|
with _patch_discovery(), _patch_single_discovery():
|
|
await async_setup_component(hass, tplink.DOMAIN, {tplink.DOMAIN: {}})
|
|
await hass.async_block_till_done()
|
|
assert already_migrated_config_entry.state == ConfigEntryState.LOADED
|
|
await hass.config_entries.async_unload(already_migrated_config_entry.entry_id)
|
|
await hass.async_block_till_done()
|
|
assert already_migrated_config_entry.state == ConfigEntryState.NOT_LOADED
|
|
|
|
|
|
async def test_config_entry_retry(hass):
|
|
"""Test that a config entry can be retried."""
|
|
already_migrated_config_entry = MockConfigEntry(
|
|
domain=DOMAIN, data={CONF_HOST: IP_ADDRESS}, unique_id=MAC_ADDRESS
|
|
)
|
|
already_migrated_config_entry.add_to_hass(hass)
|
|
with _patch_discovery(no_device=True), _patch_single_discovery(no_device=True):
|
|
await async_setup_component(hass, tplink.DOMAIN, {tplink.DOMAIN: {}})
|
|
await hass.async_block_till_done()
|
|
assert already_migrated_config_entry.state == ConfigEntryState.SETUP_RETRY
|