mirror of
https://github.com/home-assistant/core.git
synced 2025-05-01 20:57:51 +00:00
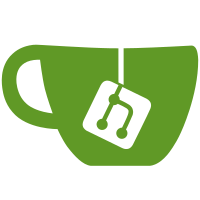
So far the Flo integration only supports shutoff valves. Add support for Flo leak detector pucks, which measure temperature and humidity in addition to providing leak alerts.
54 lines
2.0 KiB
Python
54 lines
2.0 KiB
Python
"""Test Flo by Moen sensor entities."""
|
|
from homeassistant.components.flo.const import DOMAIN as FLO_DOMAIN
|
|
from homeassistant.const import ATTR_ENTITY_ID, CONF_PASSWORD, CONF_USERNAME
|
|
from homeassistant.setup import async_setup_component
|
|
|
|
from .common import TEST_PASSWORD, TEST_USER_ID
|
|
|
|
|
|
async def test_sensors(hass, config_entry, aioclient_mock_fixture):
|
|
"""Test Flo by Moen sensors."""
|
|
config_entry.add_to_hass(hass)
|
|
assert await async_setup_component(
|
|
hass, FLO_DOMAIN, {CONF_USERNAME: TEST_USER_ID, CONF_PASSWORD: TEST_PASSWORD}
|
|
)
|
|
await hass.async_block_till_done()
|
|
|
|
assert len(hass.data[FLO_DOMAIN][config_entry.entry_id]["devices"]) == 2
|
|
|
|
# we should have 5 entities for the valve
|
|
assert hass.states.get("sensor.current_system_mode").state == "home"
|
|
assert hass.states.get("sensor.today_s_water_usage").state == "3.7"
|
|
assert hass.states.get("sensor.water_flow_rate").state == "0"
|
|
assert hass.states.get("sensor.water_pressure").state == "54.2"
|
|
assert hass.states.get("sensor.water_temperature").state == "21"
|
|
|
|
# and 3 entities for the detector
|
|
assert hass.states.get("sensor.temperature").state == "16"
|
|
assert hass.states.get("sensor.humidity").state == "43"
|
|
assert hass.states.get("sensor.battery").state == "100"
|
|
|
|
|
|
async def test_manual_update_entity(
|
|
hass, config_entry, aioclient_mock_fixture, aioclient_mock
|
|
):
|
|
"""Test manual update entity via service homeasasistant/update_entity."""
|
|
config_entry.add_to_hass(hass)
|
|
assert await async_setup_component(
|
|
hass, FLO_DOMAIN, {CONF_USERNAME: TEST_USER_ID, CONF_PASSWORD: TEST_PASSWORD}
|
|
)
|
|
await hass.async_block_till_done()
|
|
|
|
assert len(hass.data[FLO_DOMAIN][config_entry.entry_id]["devices"]) == 2
|
|
|
|
await async_setup_component(hass, "homeassistant", {})
|
|
|
|
call_count = aioclient_mock.call_count
|
|
await hass.services.async_call(
|
|
"homeassistant",
|
|
"update_entity",
|
|
{ATTR_ENTITY_ID: ["sensor.current_system_mode"]},
|
|
blocking=True,
|
|
)
|
|
assert aioclient_mock.call_count == call_count + 2
|