mirror of
https://github.com/home-assistant/core.git
synced 2025-04-25 17:57:55 +00:00
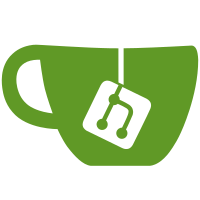
* Migrate from homeconnect dependency to aiohomeconnect * Reload the integration if there is an API error on event stream * fix typos at coordinator tests * Setup config entry at coordinator tests * fix ruff * Bump aiohomeconnect to version 0.11.4 * Fix set program options * Use context based updates at coordinator * Improved how `context_callbacks` cache is invalidated * fix * fixes and improvements at coordinator Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Remove stale Entity inheritance * Small improvement for light subscriptions * Remove non-needed function It had its purpose before some refactoring before the firs commit, no is no needed as is only used at HomeConnectEntity constructor * Static methods and variables at conftest * Refresh the data after an event stream interruption * Cleaned debug logs * Fetch programs at coordinator * Improvements Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Simplify obtaining power settings from coordinator data Co-authored-by: Martin Hjelmare <marhje52@gmail.com> * Remove unnecessary statement * use `is UNDEFINED` instead of `isinstance` * Request power setting only when it is strictly necessary * Bump aiohomeconnect to 0.12.1 * use raw keys for diagnostics * Use keyword arguments where needed * Remove unnecessary statements Co-authored-by: Martin Hjelmare <marhje52@gmail.com> --------- Co-authored-by: Martin Hjelmare <marhje52@gmail.com>
116 lines
3.9 KiB
Python
116 lines
3.9 KiB
Python
"""Provides a select platform for Home Connect."""
|
|
|
|
from typing import cast
|
|
|
|
from aiohomeconnect.model import EventKey, ProgramKey
|
|
from aiohomeconnect.model.error import HomeConnectError
|
|
|
|
from homeassistant.components.select import SelectEntity, SelectEntityDescription
|
|
from homeassistant.core import HomeAssistant
|
|
from homeassistant.exceptions import HomeAssistantError
|
|
from homeassistant.helpers.entity_platform import AddEntitiesCallback
|
|
|
|
from .const import APPLIANCES_WITH_PROGRAMS, DOMAIN, SVE_TRANSLATION_PLACEHOLDER_PROGRAM
|
|
from .coordinator import (
|
|
HomeConnectApplianceData,
|
|
HomeConnectConfigEntry,
|
|
HomeConnectCoordinator,
|
|
)
|
|
from .entity import HomeConnectEntity
|
|
from .utils import bsh_key_to_translation_key, get_dict_from_home_connect_error
|
|
|
|
TRANSLATION_KEYS_PROGRAMS_MAP = {
|
|
bsh_key_to_translation_key(program.value): cast(ProgramKey, program)
|
|
for program in ProgramKey
|
|
if program != ProgramKey.UNKNOWN
|
|
}
|
|
|
|
PROGRAMS_TRANSLATION_KEYS_MAP = {
|
|
value: key for key, value in TRANSLATION_KEYS_PROGRAMS_MAP.items()
|
|
}
|
|
|
|
PROGRAM_SELECT_ENTITY_DESCRIPTIONS = (
|
|
SelectEntityDescription(
|
|
key=EventKey.BSH_COMMON_ROOT_ACTIVE_PROGRAM,
|
|
translation_key="active_program",
|
|
),
|
|
SelectEntityDescription(
|
|
key=EventKey.BSH_COMMON_ROOT_SELECTED_PROGRAM,
|
|
translation_key="selected_program",
|
|
),
|
|
)
|
|
|
|
|
|
async def async_setup_entry(
|
|
hass: HomeAssistant,
|
|
entry: HomeConnectConfigEntry,
|
|
async_add_entities: AddEntitiesCallback,
|
|
) -> None:
|
|
"""Set up the Home Connect select entities."""
|
|
|
|
async_add_entities(
|
|
HomeConnectProgramSelectEntity(entry.runtime_data, appliance, desc)
|
|
for appliance in entry.runtime_data.data.values()
|
|
for desc in PROGRAM_SELECT_ENTITY_DESCRIPTIONS
|
|
if appliance.info.type in APPLIANCES_WITH_PROGRAMS
|
|
)
|
|
|
|
|
|
class HomeConnectProgramSelectEntity(HomeConnectEntity, SelectEntity):
|
|
"""Select class for Home Connect programs."""
|
|
|
|
def __init__(
|
|
self,
|
|
coordinator: HomeConnectCoordinator,
|
|
appliance: HomeConnectApplianceData,
|
|
desc: SelectEntityDescription,
|
|
) -> None:
|
|
"""Initialize the entity."""
|
|
super().__init__(
|
|
coordinator,
|
|
appliance,
|
|
desc,
|
|
)
|
|
self._attr_options = [
|
|
PROGRAMS_TRANSLATION_KEYS_MAP[program.key]
|
|
for program in appliance.programs
|
|
if program.key != ProgramKey.UNKNOWN
|
|
]
|
|
self.start_on_select = desc.key == EventKey.BSH_COMMON_ROOT_ACTIVE_PROGRAM
|
|
self._attr_current_option = None
|
|
|
|
def update_native_value(self) -> None:
|
|
"""Set the program value."""
|
|
event = self.appliance.events.get(cast(EventKey, self.bsh_key))
|
|
self._attr_current_option = (
|
|
PROGRAMS_TRANSLATION_KEYS_MAP.get(cast(ProgramKey, event.value))
|
|
if event
|
|
else None
|
|
)
|
|
|
|
async def async_select_option(self, option: str) -> None:
|
|
"""Select new program."""
|
|
program_key = TRANSLATION_KEYS_PROGRAMS_MAP[option]
|
|
try:
|
|
if self.start_on_select:
|
|
await self.coordinator.client.start_program(
|
|
self.appliance.info.ha_id, program_key=program_key
|
|
)
|
|
else:
|
|
await self.coordinator.client.set_selected_program(
|
|
self.appliance.info.ha_id, program_key=program_key
|
|
)
|
|
except HomeConnectError as err:
|
|
if self.start_on_select:
|
|
translation_key = "start_program"
|
|
else:
|
|
translation_key = "select_program"
|
|
raise HomeAssistantError(
|
|
translation_domain=DOMAIN,
|
|
translation_key=translation_key,
|
|
translation_placeholders={
|
|
**get_dict_from_home_connect_error(err),
|
|
SVE_TRANSLATION_PLACEHOLDER_PROGRAM: program_key.value,
|
|
},
|
|
) from err
|