mirror of
https://github.com/home-assistant/core.git
synced 2025-04-25 01:38:02 +00:00
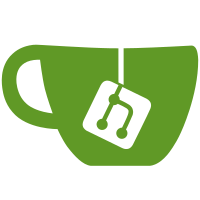
* feat: scaffold integration, configure client * feat: register services, allow API key auth flow * feat: register detection, classification services * test(viam): add test coverage * chore(viam): update viam-sdk version * fix(viam): add service schemas and translation keys * test(viam): update config flow to use new selector values * chore(viam): update viam-sdk to 0.11.0 * feat: add exceptions translation stings * refactor(viam): use constant keys, defer filesystem IO execution * fix(viam): add missing constants, resolve correct image for services * fix(viam): use lokalize string refs, resolve more constant strings * fix(viam): move service registration to async_setup * refactor: abstract services into separate module outside of manager * refactor(viam): extend common vol schemas * refactor(viam): consolidate common service values * refactor(viam): replace FlowResult with ConfigFlowResult * chore(viam): add icons.json for services * refactor(viam): use org API key to connect to robot * fix(viam): close app client if user abort config flow * refactor(viam): run ruff formatter * test(viam): confirm 100% coverage of config_flow * refactor(viam): simplify manager, clean up config flow methods * refactor(viam): split auth step into auth_api & auth_location * refactor(viam): remove use of SelectOptionDict for auth choice, update strings * fix(viam): use sentence case for translation strings * test(viam): create mock_viam_client fixture for reusable mock
61 lines
1.7 KiB
Python
61 lines
1.7 KiB
Python
"""Common fixtures for the viam tests."""
|
|
|
|
import asyncio
|
|
from collections.abc import Generator
|
|
from dataclasses import dataclass
|
|
from unittest.mock import AsyncMock, MagicMock, patch
|
|
|
|
import pytest
|
|
from viam.app.viam_client import ViamClient
|
|
|
|
|
|
@dataclass
|
|
class MockLocation:
|
|
"""Fake location for testing."""
|
|
|
|
id: str = "13"
|
|
name: str = "home"
|
|
|
|
|
|
@dataclass
|
|
class MockRobot:
|
|
"""Fake robot for testing."""
|
|
|
|
id: str = "1234"
|
|
name: str = "test"
|
|
|
|
|
|
def async_return(result):
|
|
"""Allow async return value with MagicMock."""
|
|
|
|
future = asyncio.Future()
|
|
future.set_result(result)
|
|
return future
|
|
|
|
|
|
@pytest.fixture
|
|
def mock_setup_entry() -> Generator[AsyncMock, None, None]:
|
|
"""Override async_setup_entry."""
|
|
with patch(
|
|
"homeassistant.components.viam.async_setup_entry", return_value=True
|
|
) as mock_setup_entry:
|
|
yield mock_setup_entry
|
|
|
|
|
|
@pytest.fixture(name="mock_viam_client")
|
|
def mock_viam_client_fixture() -> Generator[tuple[MagicMock, MockRobot], None, None]:
|
|
"""Override ViamClient from Viam SDK."""
|
|
with (
|
|
patch("viam.app.viam_client.ViamClient") as MockClient,
|
|
patch.object(ViamClient, "create_from_dial_options") as mock_create_client,
|
|
):
|
|
instance: MagicMock = MockClient.return_value
|
|
mock_create_client.return_value = instance
|
|
|
|
mock_location = MockLocation()
|
|
mock_robot = MockRobot()
|
|
instance.app_client.list_locations.return_value = async_return([mock_location])
|
|
instance.app_client.get_location.return_value = async_return(mock_location)
|
|
instance.app_client.list_robots.return_value = async_return([mock_robot])
|
|
yield instance, mock_robot
|