mirror of
https://github.com/home-assistant/core.git
synced 2025-07-13 08:17:08 +00:00
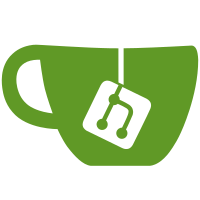
* Add Config Flow to lg_netcast * Add YAML import to Lg Netcast ConfigFlow Deprecates YAML config support * Add LG Netcast Device triggers for turn_on action * Add myself to LG Netcast codeowners * Remove unnecessary user_input validation check. * Move netcast discovery logic to the backend * Use FlowResultType Enum for tests * Mock pylgnetcast.query_device_info instead of _send_to_tv * Refactor lg_netcast client discovery, simplify YAML import * Simplify CONF_NAME to use friendly name Fix: Use Friendly name for Name * Expose model to DeviceInfo * Add test for testing YAML import when not TV not online * Switch to entity_name for LGTVDevice * Add data_description to host field in user step * Wrap try only around _get_session_id * Send regular request for access_token to ensure it display on the TV * Stop displaying access token when flow is aborted * Remove config_flow only consts and minor fixups * Simplify media_player logic & raise new migration issue * Add async_unload_entry * Create issues when import config flow fails, and raise only a single yaml deprecation issue type * Remove single use trigger helpers * Bump issue deprecation breakage version * Lint --------- Co-authored-by: Erik Montnemery <erik@montnemery.com>
60 lines
1.6 KiB
Python
60 lines
1.6 KiB
Python
"""Helper functions for LG Netcast TV."""
|
|
|
|
from typing import TypedDict
|
|
import xml.etree.ElementTree as ET
|
|
|
|
from pylgnetcast import LgNetCastClient
|
|
from requests import RequestException
|
|
|
|
from homeassistant.core import HomeAssistant, callback
|
|
from homeassistant.helpers import device_registry as dr
|
|
from homeassistant.helpers.device_registry import DeviceEntry
|
|
|
|
from .const import DOMAIN
|
|
|
|
|
|
class LGNetCastDetailDiscoveryError(Exception):
|
|
"""Unable to retrieve details from Netcast TV."""
|
|
|
|
|
|
class NetcastDetails(TypedDict):
|
|
"""Netcast TV Details."""
|
|
|
|
uuid: str
|
|
model_name: str
|
|
friendly_name: str
|
|
|
|
|
|
async def async_discover_netcast_details(
|
|
hass: HomeAssistant, client: LgNetCastClient
|
|
) -> NetcastDetails:
|
|
"""Discover UUID and Model Name from Netcast Tv."""
|
|
try:
|
|
resp = await hass.async_add_executor_job(client.query_device_info)
|
|
except RequestException as err:
|
|
raise LGNetCastDetailDiscoveryError(
|
|
f"Error in connecting to {client.url}"
|
|
) from err
|
|
except ET.ParseError as err:
|
|
raise LGNetCastDetailDiscoveryError("Invalid XML") from err
|
|
|
|
if resp is None:
|
|
raise LGNetCastDetailDiscoveryError("Empty response received")
|
|
|
|
return resp
|
|
|
|
|
|
@callback
|
|
def async_get_device_entry_by_device_id(
|
|
hass: HomeAssistant, device_id: str
|
|
) -> DeviceEntry:
|
|
"""Get Device Entry from Device Registry by device ID.
|
|
|
|
Raises ValueError if device ID is invalid.
|
|
"""
|
|
device_reg = dr.async_get(hass)
|
|
if (device := device_reg.async_get(device_id)) is None:
|
|
raise ValueError(f"Device {device_id} is not a valid {DOMAIN} device.")
|
|
|
|
return device
|