mirror of
https://github.com/home-assistant/core.git
synced 2025-07-13 08:17:08 +00:00
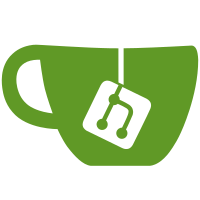
* Add circular mean statistics * fixes * Add has_circular_mean and fix tests * Fix mypy * Rename to MEASUREMENT_ANGLE * Fix kitchen_sink tests * Fix sensor tests * for testing only * Revert ws command change * Apply suggestions * test only * add custom handling for postgres * fix recursion limit * Check if column is already available * Set default false and not nullable for has_circular_mean * Proper fix to be backwards compatible * Fix value is None * Align with schema * Remove has_circular_mean from test schemas as it's not required anymore * fix wrong column type * Use correct variable to reduce stats * Add guard that the uom is matching a valid one from the state class * Add some tests * Fix tests again * Use mean_type in StatisticsMetato difference between different mean type algorithms * Fix leftovers * Fix kitchen_sink tests * Fix postgres * Add circular mean test * Add mean_type_changed stats issue * Align the attributes with unit_changed * Fix mean_type_change stats issue * Add missing sensor recorder tests * Add test_statistic_during_period_circular_mean * Add mean_weight * Add test_statistic_during_period_hole_circular_mean * Use seperate migration step to null has_mean * Typo ARITHMETIC * Implement requested changes * Implement requested changes * Split into #141444 * Add StatisticMeanType.NONE and forbid that mean_type can be None * Fix mean_type * Implement requested changes * Small leftover of latest StatisticMeanType changes
85 lines
2.5 KiB
Python
85 lines
2.5 KiB
Python
"""Recorder constants."""
|
|
|
|
from __future__ import annotations
|
|
|
|
from enum import StrEnum
|
|
from typing import TYPE_CHECKING
|
|
|
|
from homeassistant.const import (
|
|
ATTR_ATTRIBUTION,
|
|
ATTR_RESTORED,
|
|
ATTR_SUPPORTED_FEATURES,
|
|
EVENT_RECORDER_5MIN_STATISTICS_GENERATED, # noqa: F401
|
|
EVENT_RECORDER_HOURLY_STATISTICS_GENERATED, # noqa: F401
|
|
)
|
|
from homeassistant.helpers.json import JSON_DUMP # noqa: F401
|
|
|
|
if TYPE_CHECKING:
|
|
from .core import Recorder # noqa: F401
|
|
|
|
|
|
SQLITE_URL_PREFIX = "sqlite://"
|
|
MARIADB_URL_PREFIX = "mariadb://"
|
|
MARIADB_PYMYSQL_URL_PREFIX = "mariadb+pymysql://"
|
|
MYSQLDB_URL_PREFIX = "mysql://"
|
|
MYSQLDB_PYMYSQL_URL_PREFIX = "mysql+pymysql://"
|
|
DOMAIN = "recorder"
|
|
|
|
CONF_DB_INTEGRITY_CHECK = "db_integrity_check"
|
|
|
|
MAX_QUEUE_BACKLOG_MIN_VALUE = 65000
|
|
MIN_AVAILABLE_MEMORY_FOR_QUEUE_BACKLOG = 256 * 1024**2
|
|
|
|
# As soon as we have more than 999 ids, split the query as the
|
|
# MySQL optimizer handles it poorly and will no longer
|
|
# do an index only scan with a group-by
|
|
# https://github.com/home-assistant/core/issues/132865#issuecomment-2543160459
|
|
MAX_IDS_FOR_INDEXED_GROUP_BY = 999
|
|
|
|
# The maximum number of rows (events) we purge in one delete statement
|
|
|
|
DEFAULT_MAX_BIND_VARS = 4000
|
|
|
|
DB_WORKER_PREFIX = "DbWorker"
|
|
|
|
ALL_DOMAIN_EXCLUDE_ATTRS = {ATTR_ATTRIBUTION, ATTR_RESTORED, ATTR_SUPPORTED_FEATURES}
|
|
|
|
ATTR_KEEP_DAYS = "keep_days"
|
|
ATTR_REPACK = "repack"
|
|
ATTR_APPLY_FILTER = "apply_filter"
|
|
|
|
KEEPALIVE_TIME = 30
|
|
|
|
CONTEXT_ID_AS_BINARY_SCHEMA_VERSION = 36
|
|
EVENT_TYPE_IDS_SCHEMA_VERSION = 37
|
|
STATES_META_SCHEMA_VERSION = 38
|
|
LAST_REPORTED_SCHEMA_VERSION = 43
|
|
CIRCULAR_MEAN_SCHEMA_VERSION = 49
|
|
|
|
LEGACY_STATES_EVENT_ID_INDEX_SCHEMA_VERSION = 28
|
|
LEGACY_STATES_EVENT_FOREIGN_KEYS_FIXED_SCHEMA_VERSION = 43
|
|
# https://github.com/home-assistant/core/pull/120779
|
|
# fixed the foreign keys in the states table but it did
|
|
# not bump the schema version which means only databases
|
|
# created with schema 44 and later do not need the rebuild.
|
|
|
|
INTEGRATION_PLATFORM_COMPILE_STATISTICS = "compile_statistics"
|
|
INTEGRATION_PLATFORM_LIST_STATISTIC_IDS = "list_statistic_ids"
|
|
INTEGRATION_PLATFORM_UPDATE_STATISTICS_ISSUES = "update_statistics_issues"
|
|
INTEGRATION_PLATFORM_VALIDATE_STATISTICS = "validate_statistics"
|
|
|
|
INTEGRATION_PLATFORM_METHODS = {
|
|
INTEGRATION_PLATFORM_COMPILE_STATISTICS,
|
|
INTEGRATION_PLATFORM_LIST_STATISTIC_IDS,
|
|
INTEGRATION_PLATFORM_UPDATE_STATISTICS_ISSUES,
|
|
INTEGRATION_PLATFORM_VALIDATE_STATISTICS,
|
|
}
|
|
|
|
|
|
class SupportedDialect(StrEnum):
|
|
"""Supported dialects."""
|
|
|
|
SQLITE = "sqlite"
|
|
MYSQL = "mysql"
|
|
POSTGRESQL = "postgresql"
|