mirror of
https://github.com/balena-io/etcher.git
synced 2025-07-15 23:36:32 +00:00
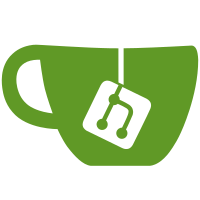
- upgrade pretty_bytes to 6.1.1 - upgrade electron-remote to 2.1.0 - upgrade semver to 7.5.4 + @types/semver to 7.5.6 - upgrade chai to 4.3.11 + @types/chai to 4.3.10 - upgrade mocha to 10.2.0 + @types/mocha to 10.0.6 - upgrade sinon to 17.0.1 + @types/sinon to 17.0.2 - remove useless @types - upgrade @svgr/webpack to 8.1.0 - upgrade @sentry/electron to 4.15.1 - upgrade tslib to 2.6.2 - upgrade immutable to 4.3.4 - upgrade redux to 4.2.1 - upgrade ts-node to 10.9.2 & ts-loader to 9.5.1 - remove mini-css-extract-plugin - upgrade husky to 8.0.3 - upgrade uuid to 9.0.1 - upgrade lint-staged to 15.2.1 - upgrade @types/node to 18.11.9 - upgrade @fortawesome/fontawesome-free to 6.5.1 - upgrade i18next to 23.7.8 & react-i18next to 11.18.6 - bump react, react-dom + related @types to 17.0.2 and rendition to 35.1.0 - fix getuid for ts - fix @types/react being in wrong deps - upgrade @types/tmp to 0.2.6 - upgrade typescript to 5.3.3 - upgrade @types/mime-types to 2.1.4 - remove d3 from deps - upgrade electron-updater to 6.1.7 - upgrade rendition to 35.1.2 - upgrade node-ipc to 9.2.3 - upgrade @types/node-ipc to 9.2.3 - upgrade electron to 27.1.3 - upgrade @electron-forge/* to 7.2.0 - upgrade @reforged/marker-appimage to 3.3.2 - upgrade style-loader to 3.3.3 - upgrade balena-lint to 7.2.4 - run CI with node 18.19 - add xxhash-addon to sidecar assets Change-type: patch
131 lines
3.4 KiB
TypeScript
131 lines
3.4 KiB
TypeScript
/*
|
||
* Copyright 2017 balena.io
|
||
*
|
||
* Licensed under the Apache License, Version 2.0 (the "License")
|
||
* you may not use this file except in compliance with the License.
|
||
* You may obtain a copy of the License at
|
||
*
|
||
* http://www.apache.org/licenses/LICENSE-2.0
|
||
*
|
||
* Unless required by applicable law or agreed to in writing, software
|
||
* distributed under the License is distributed on an "AS IS" BASIS,
|
||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
||
* See the License for the specific language governing permissions and
|
||
* limitations under the License.
|
||
*/
|
||
|
||
import type { Configuration, ModuleOptions } from 'webpack';
|
||
|
||
import {
|
||
BannerPlugin,
|
||
IgnorePlugin,
|
||
NormalModuleReplacementPlugin,
|
||
} from 'webpack';
|
||
|
||
interface ReplacementRule {
|
||
search: string;
|
||
replace: string | (() => string);
|
||
}
|
||
|
||
function slashOrAntislash(pattern: RegExp): RegExp {
|
||
return new RegExp(pattern.source.replace(/\\\//g, '(\\/|\\\\)'));
|
||
}
|
||
|
||
function replace(test: RegExp, ...replacements: ReplacementRule[]) {
|
||
return {
|
||
loader: 'string-replace-loader',
|
||
// Handle windows path separators
|
||
test: slashOrAntislash(test),
|
||
options: { multiple: replacements.map((r) => ({ ...r, strict: true })) },
|
||
};
|
||
}
|
||
|
||
const rules: Required<ModuleOptions>['rules'] = [
|
||
// Add support for native node modules
|
||
{
|
||
// We're specifying native_modules in the test because the asset relocator loader generates a
|
||
// "fake" .node file which is really a cjs file.
|
||
test: /native_modules[/\\].+\.node$/,
|
||
use: 'node-loader',
|
||
},
|
||
{
|
||
test: /[/\\]node_modules[/\\].+\.(m?js|node)$/,
|
||
parser: { amd: false },
|
||
use: {
|
||
loader: '@vercel/webpack-asset-relocator-loader',
|
||
options: {
|
||
outputAssetBase: 'native_modules',
|
||
},
|
||
},
|
||
},
|
||
{
|
||
test: /\.tsx?$/,
|
||
exclude: /(node_modules|\.webpack)/,
|
||
use: {
|
||
loader: 'ts-loader',
|
||
options: {
|
||
transpileOnly: true,
|
||
},
|
||
},
|
||
},
|
||
{
|
||
test: /\.css$/,
|
||
use: ['style-loader', 'css-loader'],
|
||
},
|
||
{
|
||
test: /\.(woff|woff2|eot|ttf|otf)$/,
|
||
loader: 'file-loader',
|
||
},
|
||
{
|
||
test: /\.svg$/,
|
||
use: '@svgr/webpack',
|
||
},
|
||
// force axios to use http backend (not xhr) to support streams
|
||
replace(/node_modules\/axios\/lib\/defaults\.js$/, {
|
||
search: './adapters/xhr',
|
||
replace: './adapters/http',
|
||
}),
|
||
];
|
||
|
||
export const rendererConfig: Configuration = {
|
||
module: {
|
||
rules,
|
||
},
|
||
plugins: [
|
||
// Force axios to use http.js, not xhr.js as we need stream support
|
||
// (its package.json file replaces http with xhr for browser targets).
|
||
new NormalModuleReplacementPlugin(
|
||
slashOrAntislash(/node_modules\/axios\/lib\/adapters\/xhr\.js/),
|
||
'./http.js',
|
||
),
|
||
// Ignore `aws-crt` which is a dependency of (ultimately) `aws4-axios` which is used
|
||
// by etcher-sdk and does a runtime check to its availability. We’re not currently
|
||
// using the “assume role” functionality (AFAIU) of aws4-axios and we don’t care that
|
||
// it’s not found, so force webpack to ignore the import.
|
||
// See https://github.com/aws/aws-sdk-js-v3/issues/3025
|
||
new IgnorePlugin({
|
||
resourceRegExp: /^aws-crt$/,
|
||
}),
|
||
// Remove "Download the React DevTools for a better development experience" message
|
||
new BannerPlugin({
|
||
banner: '__REACT_DEVTOOLS_GLOBAL_HOOK__ = { isDisabled: true };',
|
||
raw: true,
|
||
}),
|
||
],
|
||
resolve: {
|
||
extensions: ['.js', '.ts', '.jsx', '.tsx', '.css'],
|
||
},
|
||
};
|
||
|
||
export const mainConfig: Configuration = {
|
||
entry: {
|
||
etcher: './lib/gui/etcher.ts',
|
||
},
|
||
module: {
|
||
rules,
|
||
},
|
||
resolve: {
|
||
extensions: ['.js', '.ts', '.jsx', '.tsx', '.css', '.json'],
|
||
},
|
||
};
|