mirror of
https://github.com/balena-io/etcher.git
synced 2025-04-19 12:57:16 +00:00
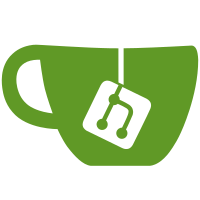
When installing balena-etcher via apt on Debian/Ubuntu, the command `balena-etcher-electron` fails with the error: line 3: /usr/bin/balena-etcher-electron.bin: No such file or directory This is because the /usr/bin/balena-etcher-electron is a symlink to /opt/balenaEtcher/balena-etcher-electron, but the script looks for balena-etcher-electron.bin in the symlink directory, not the actual script location directory. This commit uses `$(dirname "$(readlink -f "${BASH_SOURCE[0]}")")` to find the real location of the balena-etcher-electron script without symlink, so that balena-etcher-electron.bin is correctly found. Change-Type: patch Changelog-Entry: Fix error when launching from terminal when installed via apt. Fixes: https://github.com/balena-io/etcher/issues/3074
32 lines
947 B
JavaScript
32 lines
947 B
JavaScript
'use strict'
|
|
|
|
const cp = require('child_process')
|
|
const fs = require('fs')
|
|
const outdent = require('outdent')
|
|
const path = require('path')
|
|
|
|
exports.default = function(context) {
|
|
if (context.packager.platform.name !== 'linux') {
|
|
return
|
|
}
|
|
const scriptPath = path.join(context.appOutDir, context.packager.executableName)
|
|
const binPath = scriptPath + '.bin'
|
|
cp.execFileSync('mv', [scriptPath, binPath])
|
|
fs.writeFileSync(
|
|
scriptPath,
|
|
outdent({trimTrailingNewline: false})`
|
|
#!/bin/bash
|
|
|
|
# Resolve symlinks. Warning, readlink -f doesn't work on MacOS/BSD
|
|
script_dir="$(dirname "$(readlink -f "\${BASH_SOURCE[0]}")")"
|
|
|
|
if [[ $EUID -ne 0 ]] || [[ $ELECTRON_RUN_AS_NODE ]]; then
|
|
"\${script_dir}"/${context.packager.executableName}.bin "$@"
|
|
else
|
|
"\${script_dir}"/${context.packager.executableName}.bin "$@" --no-sandbox
|
|
fi
|
|
`
|
|
)
|
|
cp.execFileSync('chmod', ['+x', scriptPath])
|
|
}
|