mirror of
https://github.com/balena-io/etcher.git
synced 2025-05-01 10:47:18 +00:00
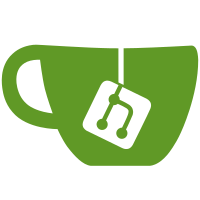
There are certain application messages that should be re-used between the CLI and the GUI. In order to allow such re-usability, we extract out the application messages used in JavaScript into `lib/shared/messages.js` as a collection of Lodash `_.template` templates. Notice this file doesn't include application messages included in Angular templates directly since it'd be hard to refactor all of them. We plan to move to React soon, which will allow moving the remaining messages very easily. Signed-off-by: Juan Cruz Viotti <jviotti@openmailbox.org>
162 lines
4.2 KiB
JavaScript
162 lines
4.2 KiB
JavaScript
/*
|
|
* Copyright 2016 resin.io
|
|
*
|
|
* Licensed under the Apache License, Version 2.0 (the "License");
|
|
* you may not use this file except in compliance with the License.
|
|
* You may obtain a copy of the License at
|
|
*
|
|
* http://www.apache.org/licenses/LICENSE-2.0
|
|
*
|
|
* Unless required by applicable law or agreed to in writing, software
|
|
* distributed under the License is distributed on an "AS IS" BASIS,
|
|
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
* See the License for the specific language governing permissions and
|
|
* limitations under the License.
|
|
*/
|
|
|
|
'use strict';
|
|
|
|
const _ = require('lodash');
|
|
const messages = require('../../../../shared/messages');
|
|
|
|
module.exports = function(
|
|
$q,
|
|
$uibModalInstance,
|
|
DrivesModel,
|
|
SelectionStateModel,
|
|
WarningModalService,
|
|
DriveConstraintsModel) {
|
|
|
|
/**
|
|
* @summary The drive selector state
|
|
* @property
|
|
* @type Object
|
|
*/
|
|
this.state = SelectionStateModel;
|
|
|
|
/**
|
|
* @summary Static methods to check a drive's properties
|
|
* @property
|
|
* @type Object
|
|
*/
|
|
this.constraints = DriveConstraintsModel;
|
|
|
|
/**
|
|
* @summary The drives model
|
|
* @property
|
|
* @type Object
|
|
*
|
|
* @description
|
|
* We expose the whole service instead of the `.drives`
|
|
* property, which is the one we're interested in since
|
|
* this allows the property to be automatically updated
|
|
* when `DrivesModel` detects a change in the drives.
|
|
*/
|
|
this.drives = DrivesModel;
|
|
|
|
/**
|
|
* @summary Determine if we can change a drive's selection state
|
|
* @function
|
|
* @private
|
|
*
|
|
* @param {Object} drive - drive
|
|
* @returns {Promise}
|
|
*
|
|
* @example
|
|
* DriveSelectorController.shouldChangeDriveSelectionState(drive)
|
|
* .then((shouldChangeDriveSelectionState) => {
|
|
* if (shouldChangeDriveSelectionState) doSomething();
|
|
* });
|
|
*/
|
|
const shouldChangeDriveSelectionState = (drive) => {
|
|
if (!DriveConstraintsModel.isDriveValid(drive, SelectionStateModel.getImage())) {
|
|
return $q.resolve(false);
|
|
}
|
|
|
|
if (DriveConstraintsModel.isDriveSizeRecommended(drive, SelectionStateModel.getImage())) {
|
|
return $q.resolve(true);
|
|
}
|
|
|
|
return WarningModalService.display({
|
|
confirmationLabel: 'Yes, continue',
|
|
description: [
|
|
messages.warning.unrecommendedDriveSize({
|
|
image: SelectionStateModel.getImage(),
|
|
drive: drive
|
|
}),
|
|
'Are you sure you want to continue?'
|
|
].join(' ')
|
|
});
|
|
};
|
|
|
|
/**
|
|
* @summary Toggle a drive selection
|
|
* @function
|
|
* @public
|
|
*
|
|
* @param {Object} drive - drive
|
|
* @returns {Promise} - resolved promise
|
|
*
|
|
* @example
|
|
* DriveSelectorController.toggleDrive({
|
|
* device: '/dev/disk2',
|
|
* size: 999999999,
|
|
* name: 'Cruzer USB drive'
|
|
* });
|
|
*/
|
|
this.toggleDrive = (drive) => {
|
|
return shouldChangeDriveSelectionState(drive).then((canChangeDriveSelectionState) => {
|
|
if (canChangeDriveSelectionState) {
|
|
SelectionStateModel.toggleSetDrive(drive.device);
|
|
}
|
|
});
|
|
};
|
|
|
|
/**
|
|
* @summary Close the modal and resolve the selected drive
|
|
* @function
|
|
* @public
|
|
*
|
|
* @example
|
|
* DriveSelectorController.closeModal();
|
|
*/
|
|
this.closeModal = () => {
|
|
const selectedDrive = SelectionStateModel.getDrive();
|
|
|
|
// Sanity check to cover the case where a drive is selected,
|
|
// the drive is then unplugged from the computer and the modal
|
|
// is resolved with a non-existent drive.
|
|
if (!selectedDrive || !_.includes(this.drives.getDrives(), selectedDrive)) {
|
|
$uibModalInstance.close();
|
|
} else {
|
|
$uibModalInstance.close(selectedDrive);
|
|
}
|
|
|
|
};
|
|
|
|
/**
|
|
* @summary Select a drive and close the modal
|
|
* @function
|
|
* @public
|
|
*
|
|
* @param {Object} drive - drive
|
|
* @returns {Promise} - resolved promise
|
|
*
|
|
* @example
|
|
* DriveSelectorController.selectDriveAndClose({
|
|
* device: '/dev/disk2',
|
|
* size: 999999999,
|
|
* name: 'Cruzer USB drive'
|
|
* });
|
|
*/
|
|
this.selectDriveAndClose = (drive) => {
|
|
return shouldChangeDriveSelectionState(drive).then((canChangeDriveSelectionState) => {
|
|
if (canChangeDriveSelectionState) {
|
|
SelectionStateModel.setDrive(drive.device);
|
|
this.closeModal();
|
|
}
|
|
});
|
|
};
|
|
|
|
};
|