mirror of
https://github.com/balena-io/etcher.git
synced 2025-07-12 05:46:31 +00:00
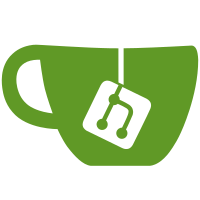
We add an icon next to the drive size that is displayed when there is a drive-image compatibility status message available. We display the first one in the list and importance is then enforced by the order they are added to the list in `drive-constraints`. Change-Type: patch Changelog-Entry: Add icon next to drive size when compatibility warnings exist.
92 lines
2.7 KiB
JavaScript
92 lines
2.7 KiB
JavaScript
/*
|
|
* Copyright 2016 resin.io
|
|
*
|
|
* Licensed under the Apache License, Version 2.0 (the "License");
|
|
* you may not use this file except in compliance with the License.
|
|
* You may obtain a copy of the License at
|
|
*
|
|
* http://www.apache.org/licenses/LICENSE-2.0
|
|
*
|
|
* Unless required by applicable law or agreed to in writing, software
|
|
* distributed under the License is distributed on an "AS IS" BASIS,
|
|
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
* See the License for the specific language governing permissions and
|
|
* limitations under the License.
|
|
*/
|
|
|
|
'use strict'
|
|
|
|
const settings = require('../../../models/settings')
|
|
const flashState = require('../../../../../shared/models/flash-state')
|
|
const analytics = require('../../../modules/analytics')
|
|
const exceptionReporter = require('../../../modules/exception-reporter')
|
|
const availableDrives = require('../../../../../shared/models/available-drives')
|
|
const selectionState = require('../../../../../shared/models/selection-state')
|
|
const driveConstraints = require('../../../../../shared/drive-constraints')
|
|
|
|
module.exports = function (
|
|
TooltipModalService,
|
|
OSOpenExternalService
|
|
) {
|
|
// Expose several modules to the template for convenience
|
|
this.selection = selectionState
|
|
this.drives = availableDrives
|
|
this.state = flashState
|
|
this.settings = settings
|
|
this.external = OSOpenExternalService
|
|
this.constraints = driveConstraints
|
|
|
|
/**
|
|
* @summary Determine if the drive step should be disabled
|
|
* @function
|
|
* @public
|
|
*
|
|
* @returns {Boolean} whether the drive step should be disabled
|
|
*
|
|
* @example
|
|
* if (MainController.shouldDriveStepBeDisabled()) {
|
|
* console.log('The drive step should be disabled');
|
|
* }
|
|
*/
|
|
this.shouldDriveStepBeDisabled = () => {
|
|
return !selectionState.hasImage()
|
|
}
|
|
|
|
/**
|
|
* @summary Determine if the flash step should be disabled
|
|
* @function
|
|
* @public
|
|
*
|
|
* @returns {Boolean} whether the flash step should be disabled
|
|
*
|
|
* @example
|
|
* if (MainController.shouldFlashStepBeDisabled()) {
|
|
* console.log('The flash step should be disabled');
|
|
* }
|
|
*/
|
|
this.shouldFlashStepBeDisabled = () => {
|
|
return !selectionState.hasDrive() || this.shouldDriveStepBeDisabled()
|
|
}
|
|
|
|
/**
|
|
* @summary Display a tooltip with the selected image details
|
|
* @function
|
|
* @public
|
|
*
|
|
* @returns {Promise}
|
|
*
|
|
* @example
|
|
* MainController.showSelectedImageDetails()
|
|
*/
|
|
this.showSelectedImageDetails = () => {
|
|
analytics.logEvent('Show selected image tooltip', {
|
|
imagePath: selectionState.getImagePath()
|
|
})
|
|
|
|
return TooltipModalService.show({
|
|
title: 'Image File Name',
|
|
message: selectionState.getImagePath()
|
|
}).catch(exceptionReporter.report)
|
|
}
|
|
}
|