mirror of
https://github.com/balena-io/etcher.git
synced 2025-07-11 21:36:32 +00:00
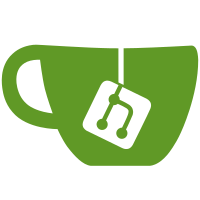
- Replace onClick arrow functions in all components that use them for efficiency reasons: 300-500% speed-up - Sort by folders and ignore case for better UX - Remove use of `rendition.Button` in files, leading to a 10-20% performance increase when browsing files - Proper sidebar width and spacing - Recents and favorites are now filtered by existence async for a tiny performance improvement - Make Breadcrumbs and Icon pure components to stop frequent re-rendering - Initial support for array constraints - Use first constraint as initial path instead of homedir if a constraint is set - Use correct design height on modal, `calc(100vh - 20px)` - Reset scroll position when browsing a new folder - Fuse Bluebird `.map()` and `.reduce()` in `files.getAllFilesMetadataAsync`. - Use `localeCompare`'s own case-insensitive option instead of calling `.toLowerCase()` twice on `n-2` files compared. - Use 16px font sizes in sidebar and files to match design. - Disable `$locationProvider.html5Mode.rewriteLinks`, which seemed to take 50ms of the directory changing time. - Leave file extension as-is in `files.getFileMetadataSync` and the async counterpart for a very minor performance improvement. Change-Type: patch
71 lines
1.7 KiB
JavaScript
71 lines
1.7 KiB
JavaScript
/*
|
|
* Copyright 2018 resin.io
|
|
*
|
|
* Licensed under the Apache License, Version 2.0 (the "License");
|
|
* you may not use this file except in compliance with the License.
|
|
* You may obtain a copy of the License at
|
|
*
|
|
* http://www.apache.org/licenses/LICENSE-2.0
|
|
*
|
|
* Unless required by applicable law or agreed to in writing, software
|
|
* distributed under the License is distributed on an "AS IS" BASIS,
|
|
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
* See the License for the specific language governing permissions and
|
|
* limitations under the License.
|
|
*/
|
|
|
|
'use strict'
|
|
|
|
const os = require('os')
|
|
const settings = require('../../../models/settings')
|
|
|
|
/* eslint-disable lodash/prefer-lodash-method */
|
|
|
|
module.exports = function (
|
|
$uibModalInstance
|
|
) {
|
|
/**
|
|
* @summary Close the modal
|
|
* @function
|
|
* @public
|
|
*
|
|
* @example
|
|
* FileSelectorController.close();
|
|
*/
|
|
this.close = () => {
|
|
$uibModalInstance.close()
|
|
}
|
|
|
|
/**
|
|
* @summary Folder to constrain the file picker to
|
|
* @function
|
|
* @public
|
|
*
|
|
* @returns {String} - folder to constrain by
|
|
*
|
|
* @example
|
|
* FileSelectorController.getFolderConstraint()
|
|
*/
|
|
this.getFolderConstraints = () => {
|
|
// TODO(Shou): get this dynamically from the mountpoint of a specific port in Etcher Pro
|
|
return settings.has('fileBrowserConstraintPath')
|
|
? settings.get('fileBrowserConstraintPath').split(',')
|
|
: []
|
|
}
|
|
|
|
/**
|
|
* @summary Get initial path
|
|
* @function
|
|
* @public
|
|
*
|
|
* @returns {String} - path
|
|
*
|
|
* @example
|
|
* <file-selector path="FileSelectorController.getPath()"></file-selector>
|
|
*/
|
|
this.getPath = () => {
|
|
const [ constraint ] = this.getFolderConstraints()
|
|
return constraint || os.homedir()
|
|
}
|
|
}
|