mirror of
https://github.com/balena-io/etcher.git
synced 2025-04-24 07:17:18 +00:00
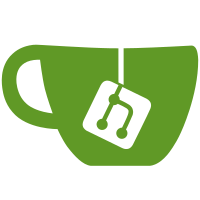
Otherwise, the `.` is interpreted as a period in a regular expression, which matches every literal character, causing some packages deep in the `node_modules/` hierarchy to be ignored for no reason. For example, if we ignore `.git`, then a package like `foo-git` will be excluded from the final package. Signed-off-by: Juan Cruz Viotti <jviottidc@gmail.com>
40 lines
982 B
JavaScript
40 lines
982 B
JavaScript
/**
|
|
* This script is in charge of building a regex of files to ignore
|
|
* when packaging for `electron-packager`'s `ignore` option.
|
|
*
|
|
* See https://github.com/electron-userland/electron-packager/blob/master/usage.txt
|
|
*
|
|
* Usage:
|
|
*
|
|
* node scripts/packageignore.js
|
|
*/
|
|
|
|
const _ = require('lodash');
|
|
const fs = require('fs');
|
|
const path = require('path');
|
|
const packageJSON = require('../package.json');
|
|
|
|
const topLevelFiles = fs.readdirSync(path.join(__dirname, '..'));
|
|
|
|
console.log(_.flatten([
|
|
packageJSON.packageIgnore,
|
|
|
|
// Development dependencies
|
|
_.map(_.keys(packageJSON.devDependencies), function(dependency) {
|
|
return path.join('node_modules', dependency);
|
|
}),
|
|
|
|
// Top level hidden files
|
|
_.map(_.filter(topLevelFiles, function(file) {
|
|
return _.startsWith(file, '.');
|
|
}), function(file) {
|
|
return '\\' + file;
|
|
}),
|
|
|
|
// Top level markdown files
|
|
_.filter(topLevelFiles, function(file) {
|
|
return _.endsWith(file, '.md');
|
|
})
|
|
|
|
]).join('|'));
|