mirror of
https://github.com/balena-io/etcher.git
synced 2025-04-24 07:17:18 +00:00
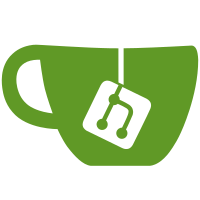
Previously dependencies weren't actually removed from `node_modules`, this runs `npm rm` on the optional dependencies, effectively excluding them, and their dependencies from the shrinkwrap file. Also the script has been hooked to the `preshrinkwrap` hook, to remove the need of having to run it manually. Change-Type: patch
45 lines
1.3 KiB
JavaScript
45 lines
1.3 KiB
JavaScript
/**
|
|
* This script is in charge of generating the `shrinkwrap` file.
|
|
*
|
|
* `npm shrinkwrap` has a bug where it will add optional dependencies
|
|
* to `npm-shrinkwrap.json`, therefore causing errors if these optional
|
|
* dependendencies are platform dependent and you then try to build
|
|
* the project in another platform.
|
|
*
|
|
* As a workaround, we keep a list of platform dependent dependencies in
|
|
* the `shrinkwrapIgnore` property of `package.json`, and manually remove
|
|
* them from `npm-shrinkwrap.json` if they exists.
|
|
*
|
|
* See: https://github.com/npm/npm/issues/2679
|
|
*/
|
|
|
|
'use strict';
|
|
|
|
const _ = require('lodash');
|
|
const path = require('path');
|
|
const packageJSON = require('../package.json');
|
|
const spawn = require('child_process').spawn;
|
|
const shrinkwrapIgnore = _.union(packageJSON.shrinkwrapIgnore, _.keys(packageJSON.optionalDependencies));
|
|
|
|
console.log('Removing:', shrinkwrapIgnore.join(', '));
|
|
|
|
/**
|
|
* Run an npm command
|
|
* @param {Array} command - list of arguments
|
|
* @returns {ChildProcess}
|
|
*/
|
|
const npm = (command) => {
|
|
return spawn('npm', command, {
|
|
cwd: path.join(__dirname, '..'),
|
|
env: process.env,
|
|
stdio: [ process.stdin, process.stdout, process.stderr ]
|
|
});
|
|
};
|
|
|
|
npm([ 'rm', '--ignore-scripts' ].concat(shrinkwrapIgnore))
|
|
.once('close', () => {
|
|
npm([ 'prune' ]).once('close', () => {
|
|
console.log('Done.');
|
|
});
|
|
});
|