mirror of
https://github.com/home-assistant/frontend.git
synced 2025-04-25 13:57:21 +00:00
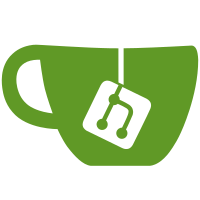
* Add build using polymer-build * Use bundle strategies to tweak stripExcludes * Only vulcanize hass.io panel * Rename hassio panel generate script * Remove hydrolysis * Get it all somewhat working * Fixes * Allow ES2015 + fix minify JS * Clarify we need to fix service worker minify * Move service worker template out of tasks folder * Fix broken CSS * Wrap it up * Fix maps
79 lines
1.9 KiB
JavaScript
Executable File
79 lines
1.9 KiB
JavaScript
Executable File
/*
|
|
TODO:
|
|
- Use gulp streams
|
|
- Use polymer bundler to vulcanize
|
|
*/
|
|
var gulp = require('gulp');
|
|
var Vulcanize = require('vulcanize');
|
|
var minify = require('html-minifier');
|
|
var fs = require('fs');
|
|
|
|
function minifyHTML(html) {
|
|
return minify.minify(html, {
|
|
customAttrAssign: [/\$=/],
|
|
removeComments: true,
|
|
removeCommentsFromCDATA: true,
|
|
removeCDATASectionsFromCDATA: true,
|
|
collapseWhitespace: true,
|
|
removeScriptTypeAttributes: true,
|
|
removeStyleLinkTypeAttributes: true,
|
|
minifyJS: true,
|
|
minifyCSS: true,
|
|
});
|
|
}
|
|
|
|
const baseVulcanOptions = {
|
|
inlineScripts: true,
|
|
inlineCss: true,
|
|
implicitStrip: true,
|
|
stripComments: true,
|
|
};
|
|
|
|
const baseExcludes = [
|
|
'bower_components/font-roboto/roboto.html',
|
|
'bower_components/paper-styles/color.html',
|
|
];
|
|
|
|
const toProcess = [
|
|
// This is the Hass.io configuration panel
|
|
// It's build standalone because it is embedded in the supervisor.
|
|
{
|
|
source: './panels/hassio/hassio-main.html',
|
|
output: './build-temp/hassio-main.html',
|
|
vulcan: new Vulcanize(Object.assign({}, baseVulcanOptions, {
|
|
stripExcludes: baseExcludes.concat([
|
|
'bower_components/polymer/polymer.html',
|
|
'bower_components/iron-meta/iron-meta.html',
|
|
]),
|
|
})),
|
|
},
|
|
];
|
|
|
|
function vulcanizeEntry(entry) {
|
|
return new Promise((resolve, reject) => {
|
|
console.log('Processing', entry.source);
|
|
entry.vulcan.process(entry.source, (err, inlinedHtml) => {
|
|
if (err !== null) {
|
|
reject(`${entry.source}: ${err}`);
|
|
return;
|
|
}
|
|
|
|
console.log('Writing', entry.output);
|
|
fs.writeFileSync(entry.output, minifyHTML(inlinedHtml));
|
|
resolve();
|
|
});
|
|
});
|
|
}
|
|
|
|
gulp.task('hassio-panel', () => {
|
|
if (!fs.existsSync('build-temp')) {
|
|
fs.mkdirSync('build-temp');
|
|
}
|
|
|
|
toProcess.reduce(
|
|
(p, entry) => p.then(() => vulcanizeEntry(entry)),
|
|
Promise.resolve());
|
|
|
|
return toProcess;
|
|
});
|