mirror of
https://github.com/home-assistant/frontend.git
synced 2025-04-22 04:17:20 +00:00
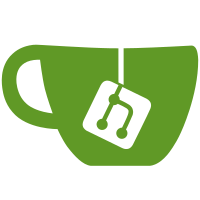
* Core POC support for polymer i18n * Move translation from core.js to html * Replace fetch with XHR * Convert translation pipeline to gulp * Convert from polyglot to Polymer localize * Pass through missing keys for custom panels * Store promise to be reused * Use cacheFirst sw handler for translations * Write full filenames to translationFingerprints * Precache en translation * Convert home-assistant-main to ES6 class * Create a localization mixin * Cleanup * Add polymer tags to annotate for linter * Rename fingerprints to translationMetadata * Build translation native names into metadata * Add language selection UI to sidebar * Provide separate message namespace argument * Store language/resources on hass object * Store translationMetadata on hass * Move language selector to config panel * Temporarily hide language selector * Small cleanups * Use dynamic-align for more flexible layout * Migrate to fetch API * Only send change events for user selection events * Update for new linting rules * Migrate build_frontend changes
59 lines
1.7 KiB
JavaScript
59 lines
1.7 KiB
JavaScript
const gulp = require('gulp');
|
|
const filter = require('gulp-filter');
|
|
const { PolymerProject, } = require('polymer-build');
|
|
const {
|
|
composeStrategies,
|
|
generateShellMergeStrategy,
|
|
} = require('polymer-bundler');
|
|
const mergeStream = require('merge-stream');
|
|
const rename = require('gulp-rename');
|
|
|
|
const polymerConfig = require('../../polymer');
|
|
|
|
const minifyStream = require('../common/transform').minifyStream;
|
|
const {
|
|
stripImportsStrategy,
|
|
stripAllButEntrypointStrategy
|
|
} = require('../common/strategy');
|
|
|
|
function renamePanel(path) {
|
|
// Rename panels to be panels/* and not their subdir
|
|
if (path.basename.substr(0, 9) === 'ha-panel-' && path.extname === '.html') {
|
|
path.dirname = 'panels/';
|
|
}
|
|
|
|
// Rename frontend
|
|
if (path.dirname === 'src' && path.basename === 'home-assistant' &&
|
|
path.extname === '.html') {
|
|
path.dirname = '';
|
|
path.basename = 'frontend';
|
|
}
|
|
}
|
|
|
|
gulp.task('build', ['ru_all', 'build-translations'], () => {
|
|
const strategy = composeStrategies([
|
|
generateShellMergeStrategy(polymerConfig.shell),
|
|
stripImportsStrategy([
|
|
'bower_components/font-roboto/roboto.html',
|
|
'bower_components/paper-styles/color.html',
|
|
]),
|
|
stripAllButEntrypointStrategy('panels/hassio/ha-panel-hassio.html')
|
|
]);
|
|
const project = new PolymerProject(polymerConfig);
|
|
|
|
return mergeStream(minifyStream(project.sources()),
|
|
minifyStream(project.dependencies()))
|
|
.pipe(project.bundler({
|
|
strategy,
|
|
strip: true,
|
|
sourcemaps: false,
|
|
stripComments: true,
|
|
inlineScripts: true,
|
|
inlineCss: true,
|
|
implicitStrip: true,
|
|
}))
|
|
.pipe(rename(renamePanel))
|
|
.pipe(filter(['**', '!src/entrypoint.html']))
|
|
.pipe(gulp.dest('build/'));
|
|
});
|