mirror of
https://github.com/home-assistant/supervisor.git
synced 2025-07-16 05:36:29 +00:00
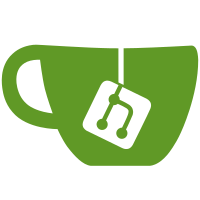
* Move repository urls to store settings file * Remove default repos from supervisor config * Fix clone at initial store load * Mock git load in repository fixture
59 lines
1.7 KiB
Python
59 lines
1.7 KiB
Python
"""Test schema validation."""
|
|
from typing import Any
|
|
|
|
import pytest
|
|
from voluptuous import Invalid
|
|
|
|
from supervisor.const import ATTR_REPOSITORIES
|
|
from supervisor.store.validate import SCHEMA_STORE_FILE, repositories
|
|
|
|
|
|
@pytest.mark.parametrize(
|
|
"config",
|
|
[
|
|
{},
|
|
{ATTR_REPOSITORIES: []},
|
|
{ATTR_REPOSITORIES: ["https://github.com/esphome/home-assistant-addon"]},
|
|
],
|
|
)
|
|
async def test_default_config(config: dict[Any]):
|
|
"""Test built-ins included by default."""
|
|
conf = SCHEMA_STORE_FILE(config)
|
|
assert ATTR_REPOSITORIES in conf
|
|
assert "core" in conf[ATTR_REPOSITORIES]
|
|
assert "local" in conf[ATTR_REPOSITORIES]
|
|
assert "https://github.com/hassio-addons/repository" in conf[ATTR_REPOSITORIES]
|
|
assert 1 == len(
|
|
[
|
|
repo
|
|
for repo in conf[ATTR_REPOSITORIES]
|
|
if repo == "https://github.com/esphome/home-assistant-addon"
|
|
]
|
|
)
|
|
|
|
|
|
@pytest.mark.parametrize(
|
|
"repo_list,valid",
|
|
[
|
|
([], True),
|
|
(["core", "local"], True),
|
|
(["https://github.com/hassio-addons/repository"], True),
|
|
(["not_a_url"], False),
|
|
(["https://fail.com/duplicate", "https://fail.com/duplicate"], False),
|
|
],
|
|
)
|
|
async def test_repository_validate(repo_list: list[str], valid: bool):
|
|
"""Test repository list validate."""
|
|
if valid:
|
|
processed = repositories(repo_list)
|
|
assert len(processed) == 4
|
|
assert set(repositories(repo_list)) == {
|
|
"core",
|
|
"local",
|
|
"https://github.com/hassio-addons/repository",
|
|
"https://github.com/esphome/home-assistant-addon",
|
|
}
|
|
else:
|
|
with pytest.raises(Invalid):
|
|
repositories(repo_list)
|