mirror of
https://github.com/home-assistant/supervisor.git
synced 2025-07-12 03:36:31 +00:00
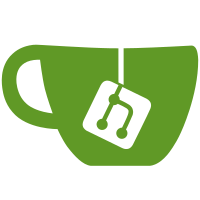
* Add udisks2 dbus support * assert mountpoints * Comment * Add reference links * docstring * fix type * fix type * add typing extensions as import * isort * additional changes * Simplify classes and conversions, fix bugs * More simplification * Fix imports * fix pip * Add additional properties and fix requirements * fix tests maybe * Handle optionality of certain configuration details * black * connect to devices before returning them * Refactor for latest dbus work * Not .items * fix mountpoints logic * use variants * Use variants for options too * isort * Switch to dbus fast * Move import to parent * Add some fixture data * Add another fixture and reduce the block devices list * Implement changes discussed with mike * Add property fixtures * update object path * Fix get_block_devices call * Tests and refactor to minimize dbus reconnects * Call super init in DBusInterfaceProxy * Fix permissions on introspection files --------- Co-authored-by: Mike Degatano <michael.degatano@gmail.com>
65 lines
1.8 KiB
Python
65 lines
1.8 KiB
Python
"""Test Docker API."""
|
|
from pathlib import Path
|
|
|
|
from aiohttp.test_utils import TestClient
|
|
import pytest
|
|
|
|
from supervisor.coresys import CoreSys
|
|
from supervisor.hardware.data import Device
|
|
|
|
|
|
@pytest.mark.asyncio
|
|
async def test_api_hardware_info(api_client: TestClient):
|
|
"""Test docker info api."""
|
|
resp = await api_client.get("/hardware/info")
|
|
result = await resp.json()
|
|
|
|
assert result["result"] == "ok"
|
|
|
|
|
|
@pytest.mark.asyncio
|
|
async def test_api_hardware_info_device(api_client: TestClient, coresys: CoreSys):
|
|
"""Test docker info api."""
|
|
coresys.hardware.update_device(
|
|
Device(
|
|
"sda",
|
|
Path("/dev/sda"),
|
|
Path("/sys/bus/usb/000"),
|
|
"sound",
|
|
None,
|
|
[Path("/dev/serial/by-id/test")],
|
|
{"ID_NAME": "xy"},
|
|
[],
|
|
)
|
|
)
|
|
|
|
resp = await api_client.get("/hardware/info")
|
|
result = await resp.json()
|
|
|
|
assert result["result"] == "ok"
|
|
assert result["data"]["devices"][-1]["name"] == "sda"
|
|
assert result["data"]["devices"][-1]["by_id"] == "/dev/serial/by-id/test"
|
|
|
|
|
|
async def test_api_hardware_info_drives(api_client: TestClient, coresys: CoreSys):
|
|
"""Test drive info."""
|
|
await coresys.dbus.udisks2.connect(coresys.dbus.bus)
|
|
|
|
resp = await api_client.get("/hardware/info")
|
|
result = await resp.json()
|
|
|
|
assert result["result"] == "ok"
|
|
assert {
|
|
drive["id"]: {fs["id"] for fs in drive["filesystems"]}
|
|
for drive in result["data"]["drives"]
|
|
} == {
|
|
"BJTD4R-0x97cde291": {
|
|
"by-id-mmc-BJTD4R_0x97cde291-part1",
|
|
"by-id-mmc-BJTD4R_0x97cde291-part3",
|
|
},
|
|
"SSK-SSK-Storage-DF56419883D56": {
|
|
"by-id-usb-SSK_SSK_Storage_DF56419883D56-0:0-part1"
|
|
},
|
|
"Generic-Flash-Disk-61BCDDB6": {"by-uuid-2802-1EDE"},
|
|
}
|